Interstitial advertising
Interstitial advertising is a full-screen ad format embedded within the app content during natural pauses, such as transitioning between game levels or completing a target action.
When the app renders an interstitial ad, the user has two options: click the ad and proceed to the advertiser site or close the ad and go back to the app.
In interstitial ads, user attention is fully focused on the ads, which is why the impression cost is higher.
Appearance
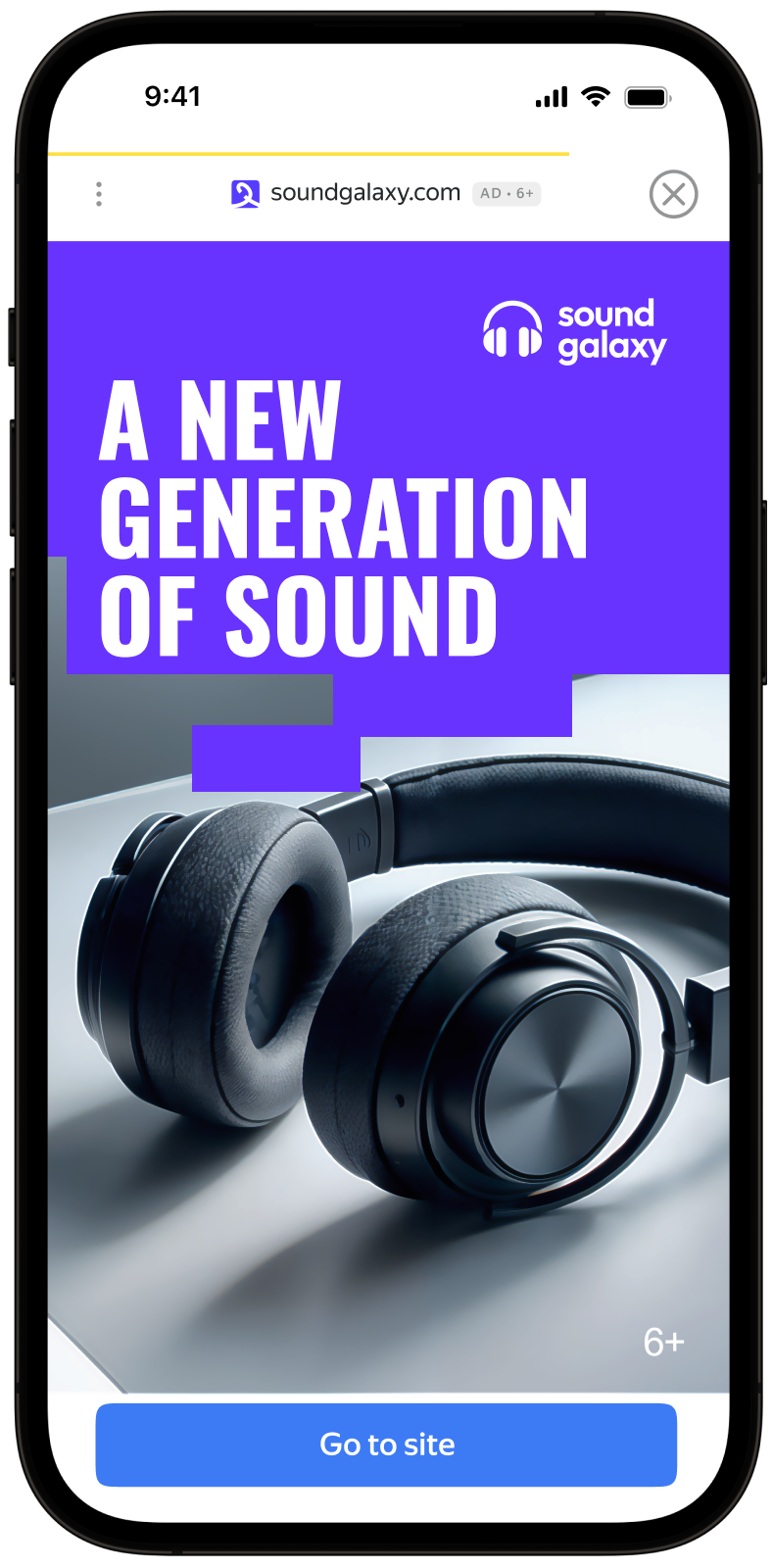
This guide will show how to integrate interstitial ads into Android apps. In addition to code examples and instructions, it contains format-specific recommendations and links to additional resources.
Prerequisite
- Follow the SDK integration steps described in Quick start.
- Initialize your ad SDK in advance.
- Make sure you're running the latest Yandex Mobile Ads SDK version. If you're using mediation, make sure you're also running the latest version of the unified build.
Implementation
Key steps for integrating interstitial ads:
- Create and configure the
InterstitialAdLoader
ad loader. - Register the listener for ad load callback methods:
InterstitialAdLoadListener
. - Load the ad.
- Pass additional settings if you're using Adfox.
- Register the
InterstitialAdEventListener
listener for ad event callback methods. - Render the
InterstitialAd
.
Features of interstitial ads integration
-
All calls to Yandex Mobile Ads SDK methods must be made from the main thread.
-
If you received an error in the
onAdFailedToLoad()
callback, don't try to load a new ad again. If there's no other option, limit the number of ad load retries. That will help avoid constant unsuccessful requests and connection issues when limitations arise. -
We recommend maintaining a strong reference to the loader and ad throughout the lifecycle of the screen that hosts interactions with the ad to avoid cleanup by the garbage cleaner.
-
We recommend using a single instance of
InterstitialAdLoader
for all ad loads to improve performance.
Loading ads
To upload interstitial ads, create and set up the InterstitialAdLoader
object.
For that, you'll need Activity or Application context.
To notify when ads load or fail to load, set the InterstitialAdLoadListener
callback method listener for the InterstitialAdLoader
object.
To load an ad, you'll need the ad unit identifier from the Partner Interface (adUnitId).
You can expand the ad request parameters through AdRequestConfiguration.Builder()
by passing user interests, contextual app data, location details, or other data. Delivering additional contextual data in the request can significantly improve your ad quality. Read more in the Ad Targeting section.
Once the ad is loaded (by calling the onAdLoaded(interstitialAd: InterstitialAd)
method), save the link to the loaded InterstitialAd
before its display is completed.
The following example shows how to load an interstitial ad from Activity:
class InterstitialAdActivity : AppCompatActivity(R.layout.activity_interstitial_ad) {
private var interstitialAd: InterstitialAd? = null
private var interstitialAdLoader: InterstitialAdLoader? = null
private lateinit var binding: ActivityInterstitialAdBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityInterstitialAdBinding.inflate(layoutInflater)
setContentView(binding.root)
// Interstitial ads loading should occur after initialization of the SDK.
// Initialize the SDK as early as possible, for example in Application.onCreate or Activity.onCreate
interstitialAdLoader = InterstitialAdLoader(this).apply {
setAdLoadListener(object : InterstitialAdLoadListener {
override fun onAdLoaded(ad: InterstitialAd) {
interstitialAd = ad
// The ad was loaded successfully. You can now show the ad.
}
override fun onAdFailedToLoad(adRequestError: AdRequestError) {
// Ad failed to load with AdRequestError.
// Attempting to load a new ad from the onAdFailedToLoad() method is strongly discouraged.
}
})
}
loadInterstitialAd()
}
private fun loadInterstitialAd() {
val adRequestConfiguration = AdRequestConfiguration.Builder("your-ad-unit-id").build()
interstitialAdLoader?.loadAd(adRequestConfiguration)
}
}
public class InterstitialAdActivity extends AppCompatActivity {
@Nullable
private InterstitialAd mInterstitialAd = null;
@Nullable
private InterstitialAdLoader mInterstitialAdLoader = null;
private ActivityInterstitialAdBinding mBinding;
public InterstitialAdActivity() {
super(R.layout.activity_interstitial_ad);
}
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mBinding = ActivityInterstitialAdBinding.inflate(getLayoutInflater());
setContentView(mBinding.getRoot());
// Interstitial ads loading should occur after initialization of the SDK.
// Initialize SDK as early as possible, for example in Application.onCreate or Activity.onCreate
mInterstitialAdLoader = new InterstitialAdLoader(this);
mInterstitialAdLoader.setAdLoadListener(new InterstitialAdLoadListener() {
@Override
public void onAdLoaded(@NonNull final InterstitialAd interstitialAd) {
mInterstitialAd = interstitialAd;
// The ad was loaded successfully. You can now show the ad.
}
@Override
public void onAdFailedToLoad(@NonNull final AdRequestError adRequestError) {
// Ad failed to load with AdRequestError.
// Attempting to load a new ad from the onAdFailedToLoad() method is strongly discouraged.
}
});
loadInterstitialAd();
}
private void loadInterstitialAd() {
if (mInterstitialAdLoader != null ) {
final AdRequestConfiguration adRequestConfiguration =
new AdRequestConfiguration.Builder("your-ad-unit-id").build();
mInterstitialAdLoader.loadAd(adRequestConfiguration);
}
}
}
Ad rendering
Interstitial ads should be displayed during natural pauses in the app's operation. One good option is inserting interstitial ads between game levels or after a conversion (for example, when a file has been downloaded).
Before rendering the ad, set the InterstitialAdEventListener
ad callback method listener.
To render an ad, you'll need to pass the Activity to the show method of the loaded ad:
private fun showAd() {
interstitialAd?.apply {
setAdEventListener(object : InterstitialAdEventListener {
override fun onAdShown() {
// Called when ad is shown.
}
override fun onAdFailedToShow(adError: AdError) {
// Called when an InterstitialAd failed to show.
// Clean resources after Ad dismissed
interstitialAd?.setAdEventListener(null)
interstitialAd = null
// Now you can preload the next interstitial ad.
loadInterstitialAd()
}
override fun onAdDismissed() {
// Called when an ad is dismissed.
// Clean resources after Ad dismissed
interstitialAd?.setAdEventListener(null)
interstitialAd = null
// Now you can preload the next interstitial ad.
loadInterstitialAd()
}
override fun onAdClicked() {
// Called when a click is recorded for an ad.
}
override fun onAdImpression(impressionData: ImpressionData?) {
// Called when an impression is recorded for an ad.
}
})
show(this@Activity)
}
}
private void showAd() {
if (mInterstitialAd != null) {
mInterstitialAd.setAdEventListener(new InterstitialAdEventListener() {
@Override
public void onAdShown() {
// Called when ad is shown.
}
@Override
public void onAdFailedToShow(@NonNull final AdError adError) {
// Called when an InterstitialAd failed to show.
}
@Override
public void onAdDismissed() {
// Called when an ad is dismissed.
// Clean resources after Ad dismissed
if (mInterstitialAd != null) {
mInterstitialAd.setAdEventListener(null);
mInterstitialAd = null;
}
// Now you can preload the next interstitial ad.
loadInterstitialAd();
}
@Override
public void onAdClicked() {
// Called when a click is recorded for an ad.
}
@Override
public void onAdImpression(@Nullable final ImpressionData impressionData) {
// Called when an impression is recorded for an ad.
}
});
mInterstitialAd.show(this);
}
}
Releasing resources
Don't store links to previously rendered ads. Call setAdEventListener(null)
for previously rendered ads.
Call setAdLoadListener(null)
for the loader if it's no longer used.
That releases the resources and prevents memory leaks.
override fun onDestroy() {
super.onDestroy()
interstitialAdLoader?.setAdLoadListener(null)
interstitialAdLoader = null
destroyInterstitialAd()
}
private fun destroyInterstitialAd() {
interstitialAd?.setAdEventListener(null)
interstitialAd = null
}
@Override
protected void onDestroy() {
super.onDestroy();
if (mInterstitialAdLoader != null) {
mInterstitialAdLoader.setAdLoadListener(null);
mInterstitialAdLoader = null;
}
destroyInterstitialAd();
}
private void destroyInterstitialAd() {
if (mInterstitialAd != null) {
mInterstitialAd.setAdEventListener(null);
mInterstitialAd = null;
}
}
Testing interstitial ad integration
Using demo ad units for ad testing
We recommend using test ads to test your interstitial ad integration and your app itself.
For guaranteed return of test ads for every ad request, we have created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-interstitial-yandex
.
Warning
Make sure that before placing the application in the store, you replaced the demo ad placement ID with a real one, obtained in Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can check your interstitial ad integration using the SDK's built-in analyzer.
The tool makes sure your interstitial ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool used for Android application debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you will see the following message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type interstitital was integrated successfully
If you're having problems integrating interstitial ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Additional resources
-
Link to GitHub.