Adaptive sticky banner
That is a small, automatically updated ad placed at the top or bottom of the app screen. It does not overlap the main app content and is often used in game apps.
Adaptive sticky banners provide maximum efficiency by optimizing the size of the ad on each device. This ad type lets developers set a maximum allowable ad width, though the optimal ad size is still determined automatically. The height of the adaptive sticky banner shouldn't exceed 15% of the screen height.
Appearance
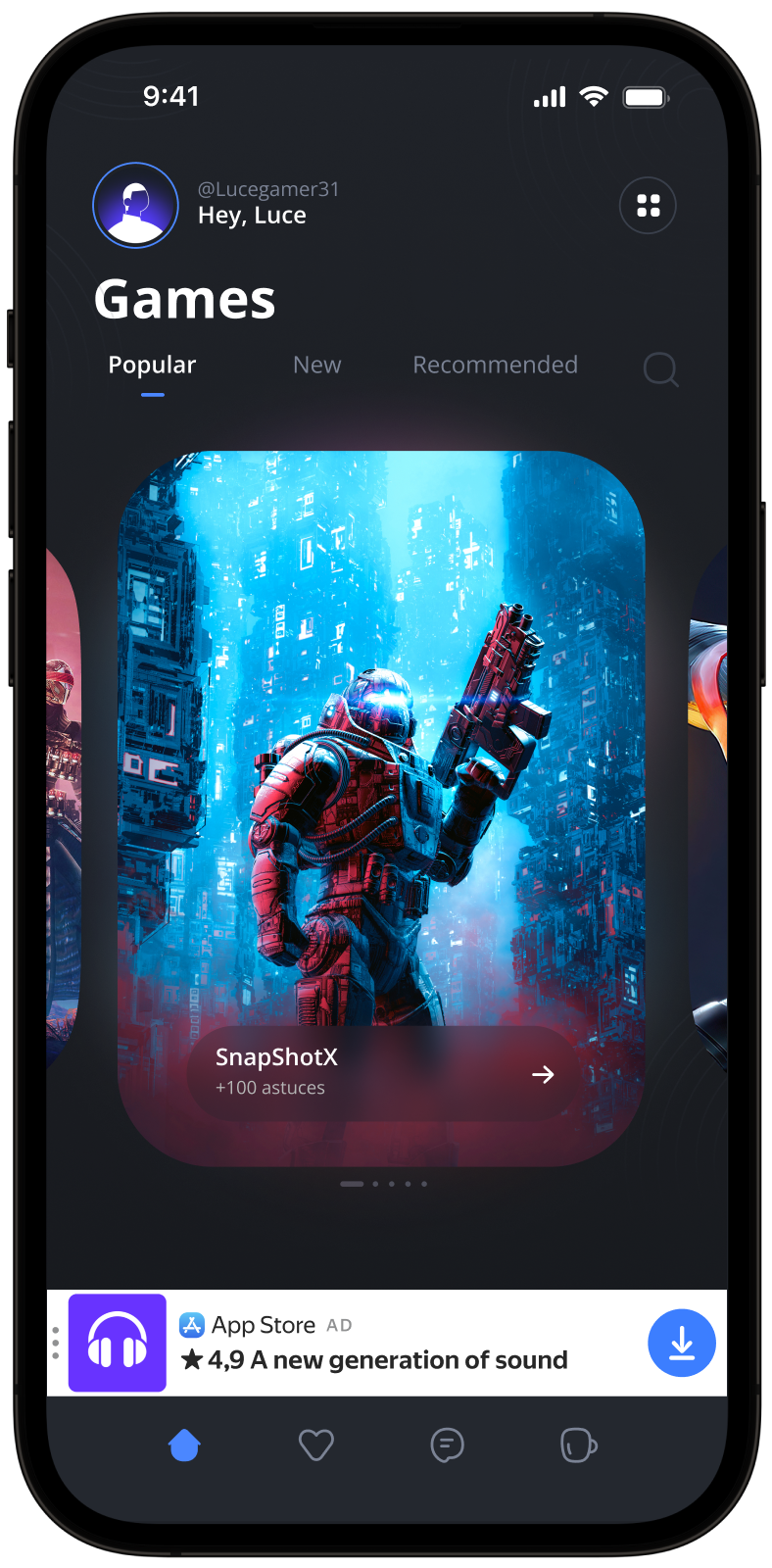
This guide shows how to integrate adaptive sticky banners into Flutter apps. In addition to code examples and instructions, it has offers format-specific recommendations and links to additional resources.
Prerequisite
- Follow the process in Quick start to integrate the Yandex Mobile Ads Flutter Plugin.
- Make sure you're running the latest Yandex Mobile Ads Flutter Plugin version. If you're using mediation, make sure you're running the latest version of the unified build.
Implementation
Key steps for integrating adaptive sticky banners:
- Create and configure a widget for displaying banner ads.
- Register a callback method listener.
- Load the ad.
Features of adaptive sticky banner integration
-
We strongly advise against attempting to load a new ad when receiving an error in the
onAdFailedToLoad()
method. If you need to load an ad fromonAdFailedToLoad()
, restrict ad load retries to avoid recurring failed ad requests due to network connection constraints. -
Adaptive sticky banners work best when using all the available width. In most cases, that's the full width of the device screen. Make sure to include all padding and safe display areas applicable to your app.
-
To get the size of the ad, use the
BannerAdSize.stickySize(adWidth)
method, which accepts the available width of the ad container as the argument. -
The
BannerAdSize
object, which is calculated using theBannerAdSize.stickySize(adWidth)
method, contains constant ad width and height values for identical devices. Once you test your app layout on a specific device, you can be sure the ad size will be constant. Use theBannerAdSize::getCalculatedBannerAdSize()
method to get the actual width and height of the ad. -
The height of the adaptive sticky banner shouldn't exceed 15% of the screen height and should be at least 50 dp.
Adding a banner widget to the app layout
To display banner ads, you need to add AdWidget
to the app layout.
Example of adding AdWidget
to the app screen layout:
@override
Widget build(BuildContext context) {
return Scaffold(
body: Align(
alignment: Alignment.bottomCenter,
child: AdWidget(bannerAd: banner),
),
);
}
Loading and rendering ads
Once AdWidget
has been created and added to the app screen, the ad needs to be loaded. The ad size must also be calculated for each device before loading an adaptive sticky banner.
That is performed automatically via the SDK API: BannerAdSize.sticky(width: screenWidth)
.
As an argument, pass the maximum permissible width of the ad container. We recommend using the entire width of the device screen or the width of the parent container. Be sure to include all the applicable paddings and safe display areas applicable to your app.
BannerAdSize getAdSize() {
final screenWidth = MediaQuery.of(context).size.width.round();
return BannerAdSize.sticky(width: screenWidth);
}
To load an ad, you'll need the ad unit ID from the Yandex Advertising Network interface (adUnitId).
To notify when ads load or fail to load and to track the life cycle of adaptive sticky banners, set callback functions when creating the BannerAd
class.
You can expand ad request parameters through AdRequest()
by passing user interests, contextual page data, location details, or other data. Delivering additional contextual data in the request can significantly improve your ad quality.
The following example shows how to load an adaptive sticky banner. After successfully loading, the banner will be displayed automatically:
class _MyHomePageState extends State<MyHomePage> {
late BannerAd banner;
var isBannerAlreadyCreated = false;
_loadAd() async {
banner = _createBanner();
setState(() {
isBannerAlreadyCreated = true;
});
// If banner was already created, you can just call
// banner.loadAd(adRequest: const AdRequest());
}
BannerAdSize _getAdSize() {
final screenWidth = MediaQuery.of(context).size.width.round();
return BannerAdSize.sticky(width: screenWidth);
}
_createBanner() {
return BannerAd(
adUnitId: 'R-M-XXXXXX-Y', // or 'demo-banner-yandex'
adSize: _getAdSize(),
adRequest: const AdRequest(),
onAdLoaded: () {
// The ad was loaded successfully. Now it will be shown.
},
onAdFailedToLoad: (error) {
// Ad failed to load with AdRequestError.
// Attempting to load a new ad from the onAdFailedToLoad() method is strongly discouraged.
},
onAdClicked: () {
// Called when a click is recorded for an ad.
},
onLeftApplication: () {
// Called when user is about to leave application (e.g., to go to the browser), as a result of clicking on the ad.
},
onReturnedToApplication: () {
// Called when user returned to application after click.
},
onImpression: (impressionData) {
// Called when an impression is recorded for an ad.
}
);
}
@override
initState() {
super.initState();
MobileAds.initialize();
_loadAd();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Align(
alignment: Alignment.bottomCenter,
child: isBannerAlreadyCreated ? AdWidget(bannerAd: banner) : null,
),
);
}
}
Releasing resources
If the callback method was called after the end of the widget lifecycle, release the resources by calling the destroy()
function on the ad object:
_createBanner() {
return BannerAd(
adUnitId: 'R-M-XXXXXX-Y', // or 'demo-banner-yandex'
adSize: getAdSize(),
adRequest: const AdRequest(),
onAdLoaded: () {
// The ad was loaded successfully. Now it will be shown.
if (!mounted) {
banner.destroy();
return;
}
},
);
}
Testing adaptive sticky banner integration
Using demo ad units for ad testing
We recommend using test ads to test your adaptive sticky banner integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-banner-yandex
.
Warning
Before publishing your app in the store, make sure to replace the demo ad placement ID with a real one obtained from the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can check your adaptive sticky banner integration using the SDK's built-in analyzer.
The tool makes sure your ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool for Android app debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you'll see this message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type banner was integrated successfully
If you're having problems integrating banner ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-banner-yandex
.
Warning
Before publishing your app to the store, make sure you replace the demo ad unit ID with a real one obtained from the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set Subsystem = com.mobile.ads.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
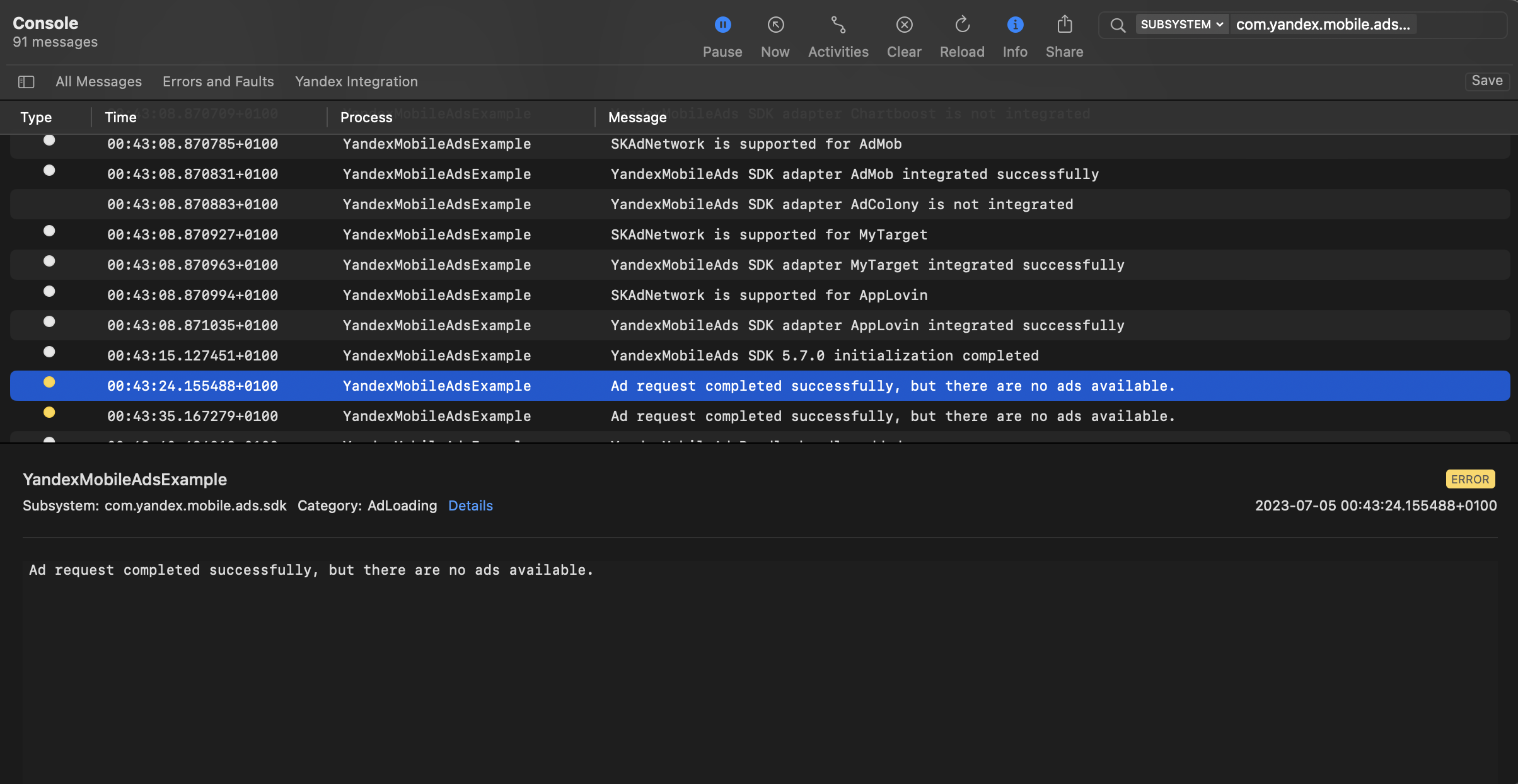
Additional resources
-
Link to pub.dev.