Rewarded ads
Rewarded ads are a popular full-screen ad format, where the user receives a reward for viewing the ad.
The display of this ad type is activated by the user, for instance, to receive a bonus or additional life in a game.
High user motivation makes this ad format the most popular and profitable in free apps.
Appearance
This guide will show how to integrate interstitial ads into a Flutter app. In addition to code examples and instructions, this guide also contains format-specific recommendations and links to additional resources.
Prerequisite
- Follow the process in Quick start to integrate the Yandex Mobile Ads Flutter Plugin.
- Make sure you're running the latest Yandex Mobile Ads Flutter Plugin version. If you're using mediation, make sure you're running the latest version of the unified build.
Implementation
Key steps for integrating rewarded ads:
- Create and configure the
RewardedAdLoader
ad loader. - Load the
RewardedAd
ad. - Register the
RewardedAdEventListener
listener for ad callback methods. - Render the
RewardedAd
. - Reward the user for viewing the ad.
Features of rewarded ad integration
- If you received an error in the
onAdFailedToLoad()
callback, don't try to load a new ad again. If there's no other option, limit the number of ad load retries. That will help avoid constant unsuccessful requests and connection issues when limitations arise.
Loading ads
To load rewarded ads, create and set up the RewardedAdLoader
object.
To do so, call the RewardedAdLoader.create(onAdLoaded, onAdFailedToLoad)
method and pass to it the loader callback listeners.
That requires the Activity context and the ad unit ID from the Yandex Advertising Network interface (adUnitId).
You can expand the set of ad request parameters via AdRequestConfiguration
, passing user interests, contextual app data, location details, or other data in the constructor. Delivering additional contextual data in the request can significantly improve your ad quality.
The following example shows how to load a rewarded ad:
late final Future<RewardedAdLoader> _adLoader;
RewardedAd? _ad;
@override
void initState() {
super.initState();
MobileAds.initialize();
_adLoader = _createRewardedAdLoader();
_loadRewardedAd();
}
Future<RewardedAdLoader> _createRewardedAdLoader() {
return RewardedAdLoader.create(
onAdLoaded: (RewardedAd rewardedAd) {
// The ad was loaded successfully. Now you can show loaded ad
_ad = rewardedAd;
},
onAdFailedToLoad: (error) {
// Ad failed to load with AdRequestError.
// Attempting to load a new ad from the onAdFailedToLoad() method is strongly discouraged.
},
);
}
Future<void> _loadRewardedAd() async {
final adLoader = await _adLoader;
await adLoader.loadAd(adRequestConfiguration: AdRequestConfiguration(adUnitId: 'R-M-XXXXXX-Y')); // For debugging, you can use 'demo-rewarded-yandex'
}
Ad rendering
Rewarded advertising is an incentive-based ad format that gives users a reward after they view the ad. The reward could be an extra life or advancing to the next level in a game. The reward format is determined at the app level.
To track the rewarded ad lifecycle and issue rewards, set the RewardedAdEventListener
callback method listener for the RewardedAd
object.
To show a rewarded ad, use the show()
method. To wait until viewing is complete, use the waitForDismiss()
method:
_showAd() async {
_ad?.setAdEventListener(
eventListener: RewardedAdEventListener(
onAdShown: () {
// Called when an ad is shown.
},
onAdFailedToShow: (error) {
// Called when an ad failed to show.
// Destroy the ad so you don't show the ad a second time.
_ad?.destroy();
_ad = null;
// Now you can preload the next ad.
_loadRewardedAd();
},
onAdClicked: () {
// Called when a click is recorded for an ad.
},
onAdDismissed: () {
// Called when ad is dismissed.
// Destroy the ad so you don't show the ad a second time.
_ad?.destroy();
_ad = null;
// Now you can preload the next ad.
_loadRewardedAd();
},
onAdImpression: (impressionData) {
// Called when an impression is recorded for an ad.
},
onRewarded: (Reward reward) {
// Called when the user can be rewarded.
}
));
await _ad?.show();
final reward = await _ad?.waitForDismiss();
if (reward != null) {
print('got ${reward.amount} of ${reward.type}');
}
}
Releasing resources
Call the destroy()
method for previously shown ads. That releases the resources and prevents memory leaks.
Don't store links to previously rendered ads.
You can use the onAdDismissed
callback method for the following actions:
_ad?.setAdEventListener(
eventListener: RewardedAdEventListener(
//...
onAdDismissed: () {
_ad?.destroy();
_ad = null;
},
//...
));
Testing the rewarded ad integration
Using demo ad units for ad testing
We recommend using test ads to test your rewarded ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-rewarded-yandex
.
Warning
Before publishing your app in the store, make sure to replace the demo ad placement ID with a real one obtained from the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your rewarded ad integration using the SDK's built-in analyzer.
The tool checks makes sure your rewarded ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool for Android app debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you'll see this message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type rewarded was integrated successfully
If you're having problems integrating rewarded ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-rewarded-yandex
.
Warning
Before publishing your app to the store, make sure you replace the demo ad unit ID with a real one obtained from the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set Subsystem = com.mobile.ads.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
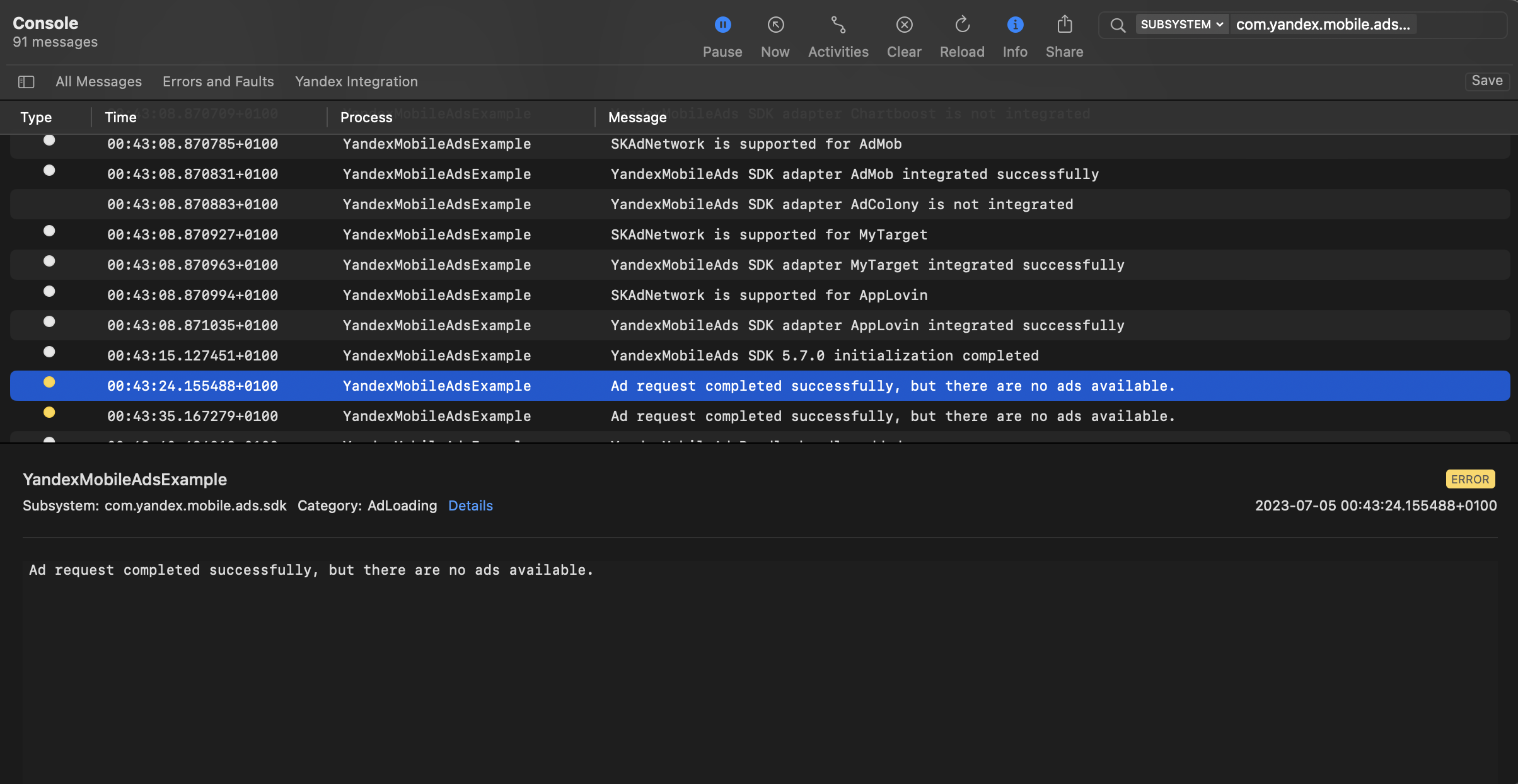
Tips
Ad preloading
Loading an ad may take several seconds, depending on the number of ad networks connected in mobile mediation and the user's internet speed. We recommend preloading ads before displaying them.
Call load
in advance to display the loaded ad at the right moment.
To start loading the next ad right after serving the current one, bind this process to the onAdDismissed()
event.
If you cache ads on too many screens that are unlikely to be shown, your ad effectiveness could drop. For example, if users complete 2-3 game levels per session, you shouldn't cache ads for 6-7 screens. Your ad viewability could decrease otherwise, and the advertising system might deprioritize your app.
To make sure you're finding a good balance for your app, track the "Share of impressions" or "Share of visible impressions" metric in the Yandex Advertising Network interface. If it's under 20%, you should probably revise your caching algorithm. The higher the percentage of impressions, the better.
Additional resources
-
Link to pub.dev.