Adaptive sticky banner
That is a small, automatically updated ad placed at the top or bottom of the app screen. It does not overlap the main app content and is often used in game apps.
Adaptive sticky banners provide maximum efficiency by optimizing the size of the ad on each device. This ad type lets developers set a maximum allowable ad width, though the optimal ad size is still determined automatically. The height of the adaptive sticky banner shouldn't exceed 15% of the screen height.
Appearance
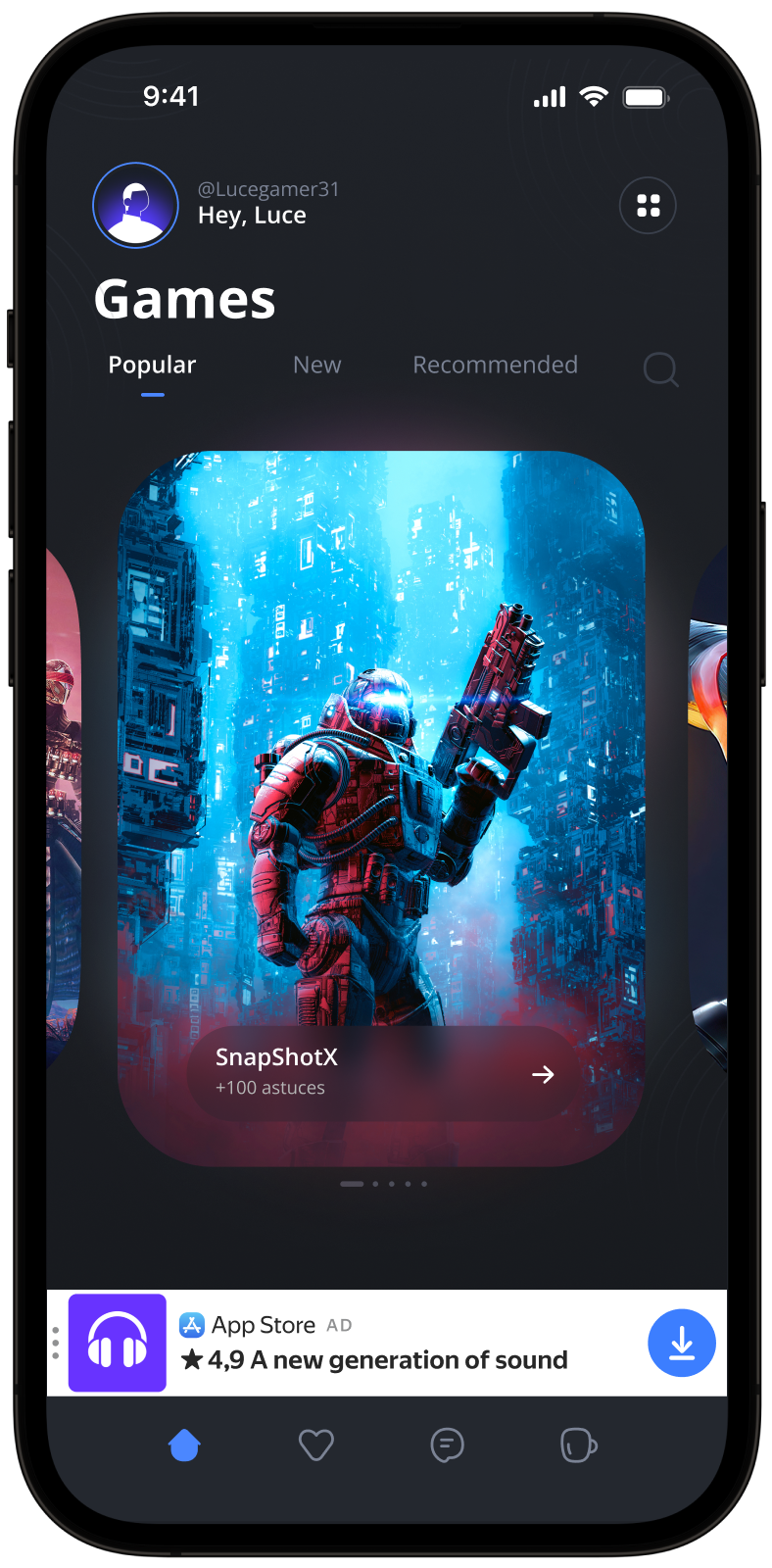
This guide shows how to integrate adaptive sticky banners into iOS apps. In addition to code examples and instructions, it contains format-specific recommendations and links to additional resources.
Prerequisite
- Follow the SDK integration steps described in Quick start.
- Initialize your ad SDK in advance.
- Make sure you're running the latest Yandex Mobile Ads SDK version. If you're using mediation, make sure you're also running the latest version of the unified build.
Implementation
Key steps for integrating adaptive sticky banners:
- Create an AdView class instance.
- Implement the delegate methods.
- Load the ad.
- Pass additional settings if you're using Adfox.
- Receive the ad in the delegate method and render it.
Features of adaptive sticky banner integration
-
All calls to Yandex Mobile Ads SDK methods must be made from the main thread.
-
We strongly advise against attempting to load a new ad when receiving an error in the
func adViewDidFailLoading(_ adView: AdView, error: Error)
delegate method. If you need to load an ad fromfunc adViewDidFailLoading(_ adView: AdView, error: Error)
, restrict ad load retries to avoid recurring failed ad requests due to network connection constraints. -
To make sure your adaptive sticky banners work correctly, use Auto Layout. Using a fixed-sized frame for the view may result in incorrect ad rendering.
-
Adaptive sticky banners work best when using all the available width. In most cases, that's the full width of the device screen. Make sure to include all padding and safe display areas applicable to your app.
-
Adaptive sticky banners are designed for placement in scrollable content. It can have the same height as the device screen or be limited to the maximum height depending on the API.
-
To get the ad size, use the
BannerAdSize.stickySize(withContainerWidth: CGFloat)
method, passing the available ad container width as an argument. -
The
BannerAdSize
object, which is calculated using theBannerAdSize.stickySize(withContainerWidth: CGFloat)
method, contains constant ad width and height values for identical devices. Once you test your app layout on a specific device, you can be sure the ad size will be constant. -
The height of the adaptive sticky banner shouldn't exceed 15% of the screen height and should be at least 50 dp.
Creating an AdView class instance
To display banner ads, create an AdView
class instance, passing the ad size and ad ID to it. You also need to define a delegate for AdView
by implementing the AdViewDelegate
protocol for your class.
Ads are calculated for devices using the BannerAdSize.stickySize(withContainerWidth:)
SDK method. As an argument, pass the maximum permissible width of the ad container. We recommend using the entire width of the device screen or the width of the parent container. Make sure you include all padding and safe display areas applicable to your app.
You will also need the advertising slot ID (adUnitId) you received in the Yandex Advertising Network interface.
Example of creating an AdView
instance in the View Controller:
final class StickyBannerViewController: UIViewController {
private lazy var adView: AdView = {
let width = view.safeAreaLayoutGuide.layoutFrame.width
let adSize = BannerAdSize.stickySize(withContainerWidth: width)
let adView = AdView(adUnitID: "R-M-XXXXX-YY", adSize: adSize)
adView.delegate = self
adView.translatesAutoresizingMaskIntoConstraints = false
return adView
}()
}
extension StickyBannerViewController: AdViewDelegate {
func adViewDidLoad(_ adView: AdView) {
// This method will call after successfully loading
}
func adViewDidFailLoading(_ adView: AdView, error: Error) {
// This method will call after getting any error while loading the ad
}
}
Loading ads
Once AdView
is created, the ad needs to be loaded.
To notify when ads load or fail to load and to track the adaptive sticky banner's life cycle, you need to set delegate properties for the AdView
class object and implement the AdViewDelegate
protocol.
You can expand ad request parameters through AdRequest
, passing user interests, contextual page data, location details, or other info. Delivering additional contextual data in the request can significantly improve your ad quality. Read more in the Ad Targeting section.
The following example shows how to load an adaptive sticky banner. After successful loading, the func adViewDidLoad(_ adView: AdView)
method of the AdViewDelegate
delegate will be called:
final class StickyBannerViewController: UIViewController {
private lazy var adView: AdView = {
let width = view.safeAreaLayoutGuide.layoutFrame.width
let adSize = BannerAdSize.stickySize(withContainerWidth: width)
let adView = AdView(adUnitID: "R-M-XXXXX-YY", adSize: adSize)
adView.delegate = self
adView.translatesAutoresizingMaskIntoConstraints = false
return adView
}()
func loadAd() {
adView.loadAd()
}
}
Displaying ads
After successfully loading the ad, you need to render it. There are two ways to do that:
Take the adView you got from the delegate method and add it to your container. Then add autolayout constraints so the banner is displayed where you want it.
final class StickyBannerViewController: UIViewController {
private lazy var adView: AdView = {
let width = view.safeAreaLayoutGuide.layoutFrame.width
let adSize = BannerAdSize.stickySize(withContainerWidth: width)
let adView = AdView(adUnitID: "R-M-XXXXX-YY", adSize: adSize)
adView.delegate = self
adView.translatesAutoresizingMaskIntoConstraints = false
return adView
}()
func showAd() {
view.addSubview(adView)
NSLayoutConstraint.activate([
adView.topAnchor.constraint(equalTo: loadButton.bottomAnchor, constant: 100),
adView.centerXAnchor.constraint(equalTo: view.centerXAnchor)
])
}
}
The banners will be added on top of all the controller's views, centered horizontally, and placed at the top or bottom of the container, respectively.
final class StickyBannerViewController: UIViewController {
private lazy var adView: AdView = {
let width = view.safeAreaLayoutGuide.layoutFrame.width
let adSize = BannerAdSize.stickySize(withContainerWidth: width)
let adView = AdView(adUnitID: "R-M-XXXXX-YY", adSize: adSize)
adView.delegate = self
adView.translatesAutoresizingMaskIntoConstraints = false
return adView
}()
func showAd() {
adView.displayAdBottom(in: view)
}
}
Testing adaptive sticky banner integration
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-banner-yandex
.
Warning
Before publishing your app to the store, make sure you replace the demo ad unit ID with a real one obtained from the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set Subsystem = com.mobile.ads.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
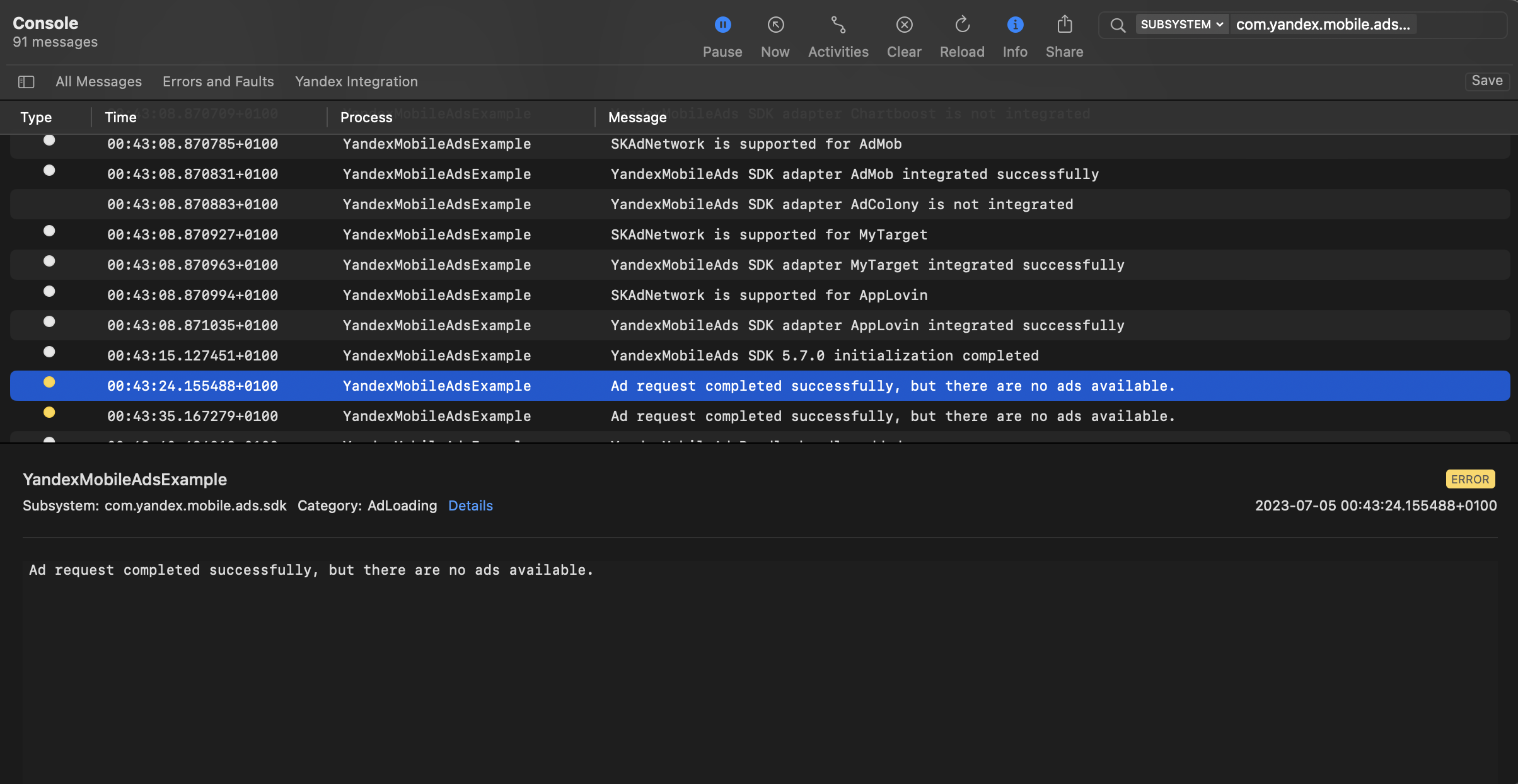
Additional resources
-
Link to GitHub.