Interstitial ads
Interstitial advertising is a full-screen ad format embedded within the app content during natural pauses, such as transitioning between game levels or completing a target action.
When the app renders an interstitial ad, the user has two options: click the ad and proceed to the advertiser site or close the ad and go back to the app.
In interstitial ads, user attention is fully focused on the ads, which is why the impression cost is higher.
Appearance
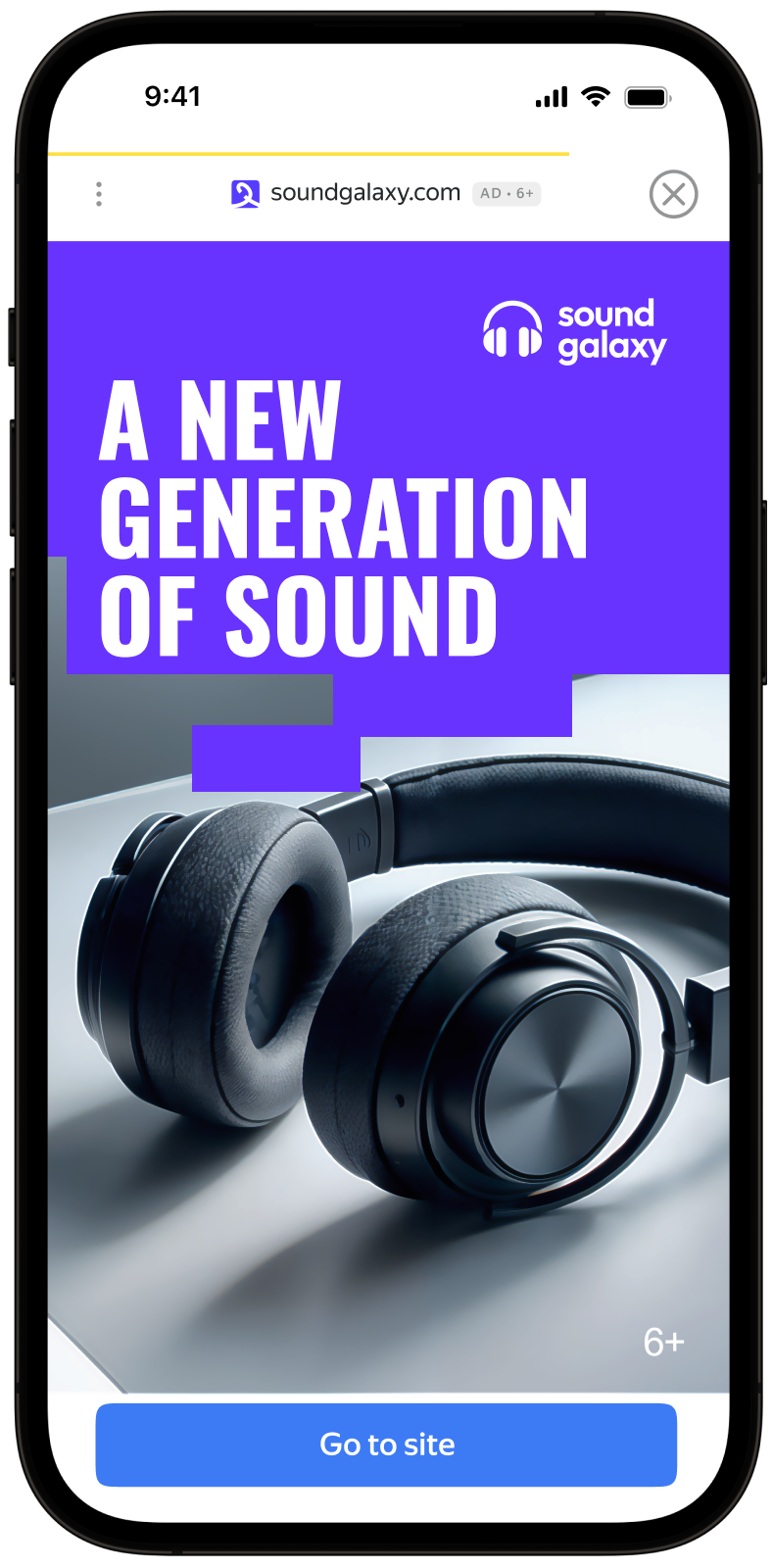
This guide will show how to integrate interstitial ads into iOS apps. In addition to code examples and instructions, it contains format-specific recommendations and links to additional resources.
Prerequisite
- Follow the SDK integration steps described in Quick start.
- Initialize your ad SDK in advance.
- Make sure you're running the latest Yandex Mobile Ads SDK version. If you're using mediation, make sure you're also running the latest version of the unified build.
Implementation
Key steps for integrating interstitial ads:
- Create and configure the
InterstitialAdLoader
ad loader. - Set a delegate and implement the required
AdInterstitialAdLoaderDelegate
methods. - Load the ad.
- Pass additional settings if you're using Adfox.
- If needed, set a delegate for the ad object and implement the required
AdInterstitialAdDelegate
methods. - Render the ad.
Features of interstitial ad integration
-
All calls to Yandex Mobile Ads SDK methods must be made from the main thread.
-
We strongly advise against attempting to load a new ad when receiving an error in the
func interstitialAdLoader(_ adLoader: InterstitialAdLoader, didFailToLoadWithError error: AdRequestError)
callback. If you need to load an ad fromfunc interstitialAdLoader(_ adLoader: InterstitialAdLoader, didFailToLoadWithError error: AdRequestError)
, restrict ad loading retries to avoid recurring failed ad requests due to network connection constraints. -
We recommend keeping a strong reference to the ad and its loader throughout the lifecycle of the screen interacting with the ad.
Loading ads
To load interstitial ads, you need to create an instance of the InterstitialAdLoader
class.
To notify when ads load or fail to load, set the delegate property for the InterstitialAdLoader
class and implement the InterstitialAdLoaderDelegate
delegate.
final class InterstitialViewController: UIViewController {
private lazy var interstitialAdLoader: InterstitialAdLoader = {
let loader = InterstitialAdLoader()
loader.delegate = self
return loader
}()
}
extension InterstitialViewController: InterstitialAdLoaderDelegate {
func interstitialAdLoader(_ adLoader: InterstitialAdLoader, didLoad interstitialAd: InterstitialAd) {
// This method will call after successfully loading
}
func interstitialAdLoader(_ adLoader: InterstitialAdLoader, didFailToLoadWithError error: AdRequestError) {
// This method will call after getting any error while loading the ad
}
}
To load an ad, you'll need the ad unit ID (adUnitId) from the Yandex Advertising Network interface.
You can expand ad request parameters through AdRequestConfiguration
, passing user interests, contextual page data, location details, or other info. Additional contextual data delivered in the request can significantly improve your ad quality. Read more in the Ad Targeting section.
The following example shows how to load an interstitial ad from the View Controller:
final class InterstitialViewController: UIViewController {
private lazy var interstitialAdLoader: InterstitialAdLoader = {
let loader = InterstitialAdLoader()
loader.delegate = self
return loader
}()
func loadAd() {
let configuration = AdRequestConfiguration(adUnitID: "R-M-XXXXX-YY")
interstitialAdLoader.loadAd(with: configuration)
}
}
Ad rendering
Interstitial ads should be displayed during natural pauses in the app's operation. One good option is inserting interstitial ads between game levels or after a conversion (for example, when a file has been downloaded).
After the interstitial ad is successfully loaded, the func interstitialAdLoader(_ adLoader: InterstitialAdLoader, didLoad interstitialAd: InterstitialAd)
method will be called. Use it to display the interstitial ad.
final class InterstitialViewController: UIViewController {
private var interstitialAd: InterstitialAd?
func showAd() {
interstitialAd?.show(from: self)
}
}
extension InterstitialViewController: InterstitialAdLoaderDelegate {
func interstitialAdLoader(_ adLoader: InterstitialAdLoader, didLoad interstitialAd: InterstitialAd) {
self.interstitialAd = interstitialAd
self.interstitialAd.delegate = self
showAd()
}
}
Testing interstitial ad integration
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-interstitial-yandex
.
Warning
Make sure that before placing the application in the store, you replaced the demo ad placement ID with a real one, obtained in the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set Subsystem = com.mobile.ads.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
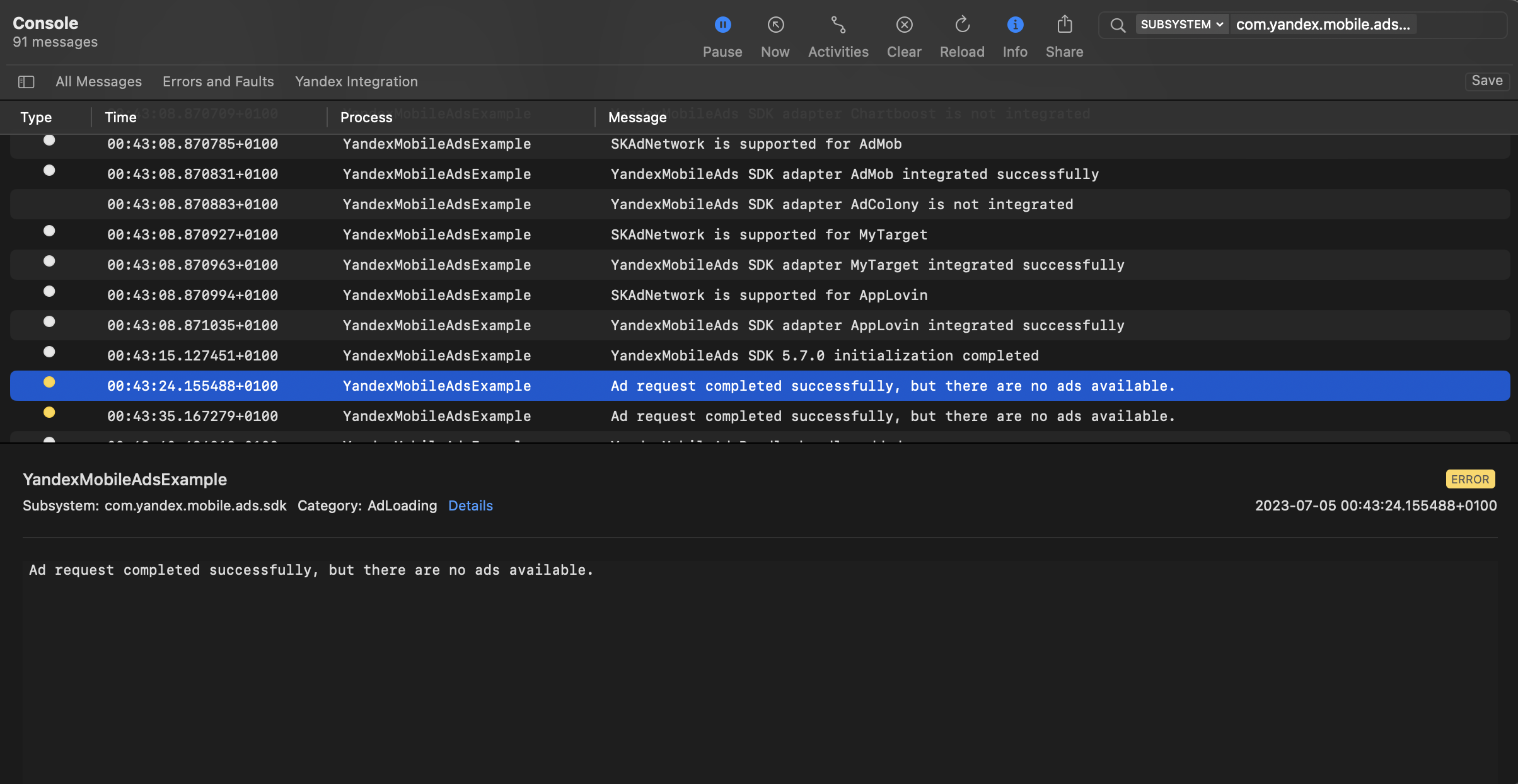
Additional resources
-
Link to GitHub.