Adaptive sticky banner
That is a small, automatically updated ad placed at the top or bottom of the app screen. It does not overlap the main app content and is often used in game apps.
Adaptive sticky banners provide maximum efficiency by optimizing the size of the ad on each device. This ad type lets developers set a maximum allowable ad width, though the optimal ad size is still determined automatically. The height of the adaptive sticky banner shouldn't exceed 15% of the screen height.
This guide covers the process of integrating adaptive sticky banners into React Native apps. Besides code samples and instructions, it also contains format-specific recommendations and links to additional resources.
Prerequisite
- Follow the Yandex Mobile Ads React Native plugin integration steps described under Quick start.
- Make sure that you have the latest version of the Yandex Mobile Ads React Native plugin. If you're using mediation, update to the most recent single build version.
Implementation
Key steps for integrating adaptive sticky banners:
- Get the banner size.
- Set up the parameters for loading ads using an
AdRequest
object. - Display the ad by setting the required properties and callback functions to handle events within the ad lifecycle.
Features of adaptive sticky banner integration
-
We strongly discourage attempting to load a new ad when receiving an error in the
onAdFailedToLoad
callback. If you need to load an ad fromonAdFailedToLoad
, limit retry attempts to avoid recurring failed ad requests in case of network connection constraints. -
For adaptive sticky banners to work properly, make your app layouts adaptive. Otherwise, your ads might render incorrectly.
-
Adaptive sticky banners work best when utilizing the full available width. In most cases, this will be the full width of the device screen. Be sure to consider the padding parameters set in your app and the display's safe area.
-
To get the size of the ad, use the method
BannerAdSize.stickySize(width)
, which accepts the available width of the ad container as an argument. -
The ad width and height values contained within a
BannerAdSize
object that was calculated using theBannerAdSize.stickySize(width)
method are consistent across the same device. When testing your app's layout on a specific device, you can be sure that the ad size for that device will remain the same. -
The height of an adaptive sticky banner never exceeds 15% of the screen height and can't be less than 50 dp.
Getting the banner size
To get the size of the ad, use the method BannerAdSize.stickySize(width)
which accepts the available width of the ad container and the maximum acceptable ad height as arguments. Be sure to consider the padding parameters set in your app and the display's safe area:
let adSize = await BannerAdSize.stickySize(Dimensions.get('window').width);
Creating an AdRequest
You can expand the ad request parameters via the AdRequest
class object. You can provide information about the user's interests, page context, location, and other additional data. Extra context added to ad requests can greatly improve the ad quality.
Ad display
To load an ad, you need the size calculated using the BannerAdSize.stickySize(width)
method as well as the placement ID that you obtained in the Partner Interface (adUnitId).
To enable notifications when ads load or fail to load and track an adaptive sticky banner's lifecycle events, set callback functions for the BannerView
component.
You can also expand the request parameters by passing the adRequest
property of the BannerView
component to the AdRequest
class object.
The example below shows how to set the adaptive inline banner properties and the callback functions for handling its lifecycle events. Once loaded, the banner is displayed automatically:
const createBanner = async () => {
let adSize = await BannerAdSize.stickySize(Dimensions.get('window').width);
let adRequest = new AdRequest({
age: '20',
contextQuery: 'context-query',
contextTags: ['context-tag'],
gender: Gender.Male,
location: new Location(55.734202, 37.588063)
});
return (
<BannerView
size={adSize}
adUnitId={'R-M-XXXXXX-Y'} // or 'demo-banner-yandex'
adRequest={adRequest}
onAdLoaded={() => console.log('Did load')}
onAdFailedToLoad={(event: any) => console.log(`Did fail to load with error: ${JSON.stringify(event.nativeEvent)}`)}
onAdClicked={() => console.log('Did click')}
onLeftApplication={() => console.log('Did leave application')}
onReturnToApplication={() => console.log('Did return to application')}
onAdImpression={(event: any) => console.log(`Did track impression: ${JSON.stringify(event.nativeEvent.impressionData)}`)}
onAdClose={() => console.log('Did close')}
/>
);
}
Testing adaptive sticky banner integration
Using demo ad units for ad testing
We recommend using test ads to test your adaptive sticky banner integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-banner-yandex
.
Warning
Before publishing your app in the store, make sure to replace the demo ad placement ID with a real one obtained from the Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can check your adaptive sticky banner integration using the SDK's built-in analyzer.
The tool makes sure your ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool for Android app debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you'll see this message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type banner was integrated successfully
If you're having problems integrating banner ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-banner-yandex
.
Warning
Before publishing your app to the store, make sure you replace the demo ad unit ID with a real one obtained from the Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set sybsystem = com.yandex.mobile.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
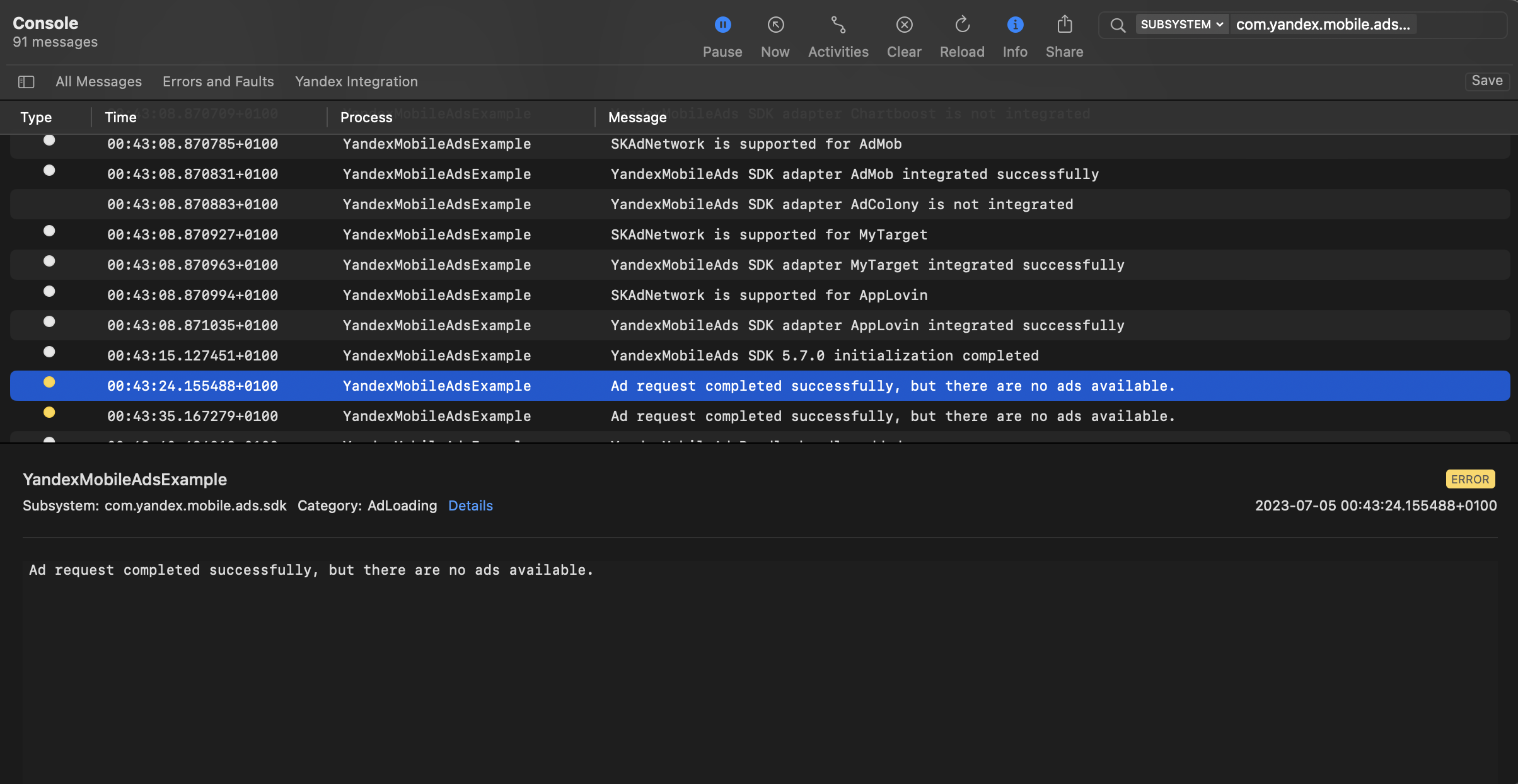
Additional resources
-
Link to GitHub.