App Open Ads
App open ads are a special ad format for monetizing your app load screens. These ads can be closed at any time and are designed to be served when users bring your app to the foreground, either at launch or when returning to it from the background.
This guide covers the process of integrating App Open Ads into React Native apps. Besides code samples and instructions, it contains format-specific recommendations and links to additional resources.
Alert
App open ads can only be placed in an app with a vertical orientation. For a horizontal orientation, ads won't be served.
Appearance
App Open Ads include a Go to the app button, which indicates to users that they're currently in your app and can close the ad. Here is an example of what this ad format may look like:
Prerequisite
- Follow the Yandex Mobile Ads React Native plugin integration steps described under Quick start.
- Make sure that you have the latest version of the Yandex Mobile Ads React Native plugin. If you're using mediation, update to the most recent single build version.
Terms
- Cold start: Launching the app when it isn't present in RAM, which creates a new app session.
- Hot start: Bringing the app from the background, where it's paused in RAM, into the foreground.
Implementation
Main steps for integrating App Open Ads:
- Create an
AppOpenAdLoader
. - Set up the parameters for loading ads using an
AdRequestConfiguration
object. - Load the
AppOpenAd
object. - Set the ad's callback functions.
- Subscribe to the app state change event using the
AppState.addEventListener('change', handleAppStateChange)
method so that you can display ads when the app is opened. - Display the
AppOpenAd
object.
Main steps
-
Create an
AppOpenAdLoader
.let loader = await InterstitialAdLoader.create() .catch((error) => { // Handle error gracefully return; });
-
Set up the parameters for loading ads using an
AdRequestConfiguration
object. To create anAdRequestConfiguration
class instance, you'll need the placement ID from the Yandex Advertising Network interface (adUnitId).You can also expand ad request parameters by providing information about the user's interests, page context, location, and other additional data when creating the
AdRequestConfiguration
object. Extra context added to ad requests can greatly improve the ad quality. Read more in the Ad Targeting section.let adRequestConfiguration = new AdRequestConfiguration( 'R-M-XXXXXX-Y', // for debug you can use 'demo-appopenad-yandex' '20', 'context-query', ['context-tag'], Gender.Female, new Location(55.734202, 37.588063) );
-
Call the
loadAd
method to load the ad.let ad = await loader.loadAd(adRequestConfiguration) .then((ad) => { // Ad loaded successfully return ad; }) .catch((error) => { // Handle error gracefully return; });
-
Set the ad's callback functions.
ad.onAdShown = () => { console.log('Did show'); }; ad.onAdFailedToShow = (error) => { console.log(`Did fail to show with error: ${JSON.stringify(error)}`); }; ad.onAdClicked = () => { console.log('Did click'); }; ad.onAdDismissed = () => { console.log('Did dismiss'); }; ad.onAdImpression = (impressionData) => { console.log(`Did track impression: ${JSON.stringify(impressionData)}`); };
-
Subscribe to the app state change event using the
AppState.addEventListener('change', handleAppStateChange)
method so that you can display ads when the app is opened. -
When the app changes to an active status, call the
show()
method to display an ad.const handleAppStateChange = (nextAppState: string) => { if (nextAppState === 'active') { ad.show(); subscription.remove(); } }; const subscription = AppState.addEventListener('change', handleAppStateChange);
Note
If the ad has already been served, calling the
show()
method will return a display error inonAdFailedToShow
.
Features of App Open Ad integration
- Ads may take a long time to load, so you should avoid increasing the cold start time if the ad hasn't loaded.
- Preload ads for subsequent hot start impressions in advance.
- We don't recommend loading App Open Ads simultaneously with other ad formats at app startup, since the app may be downloading essential operational data. Doing so could lead to excessive loads on your device and internet connection, resulting in longer ad load times.
- If the
loadAd
method returns an error, don't try to load a new ad again. If there's no other option, limit the number of ad load retries. This will help avoid constant unsuccessful requests and connection issues if there are limitations.
Testing App Open Ad integration
Using demo ad units for ad testing
We recommend using test ads to test your integration for app open ads and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-appopenad-yandex
.
Warning
Before publishing your app in the store, make sure to replace the demo ad placement ID with a real one obtained from the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can check your integration of app open ads using the SDK's built-in analyzer.
The tool makes sure your app open ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool for Android app debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you'll see this message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type App Open Ad was integrated successfully
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Using demo ad units for ad testing
We recommend using test ads to test your integration for app open ads and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-appopenad-yandex
.
Warning
Before publishing your app in the store, make sure to replace the demo ad placement ID with a real one obtained from the Yandex Advertising Network interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set Subsystem = com.mobile.ads.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
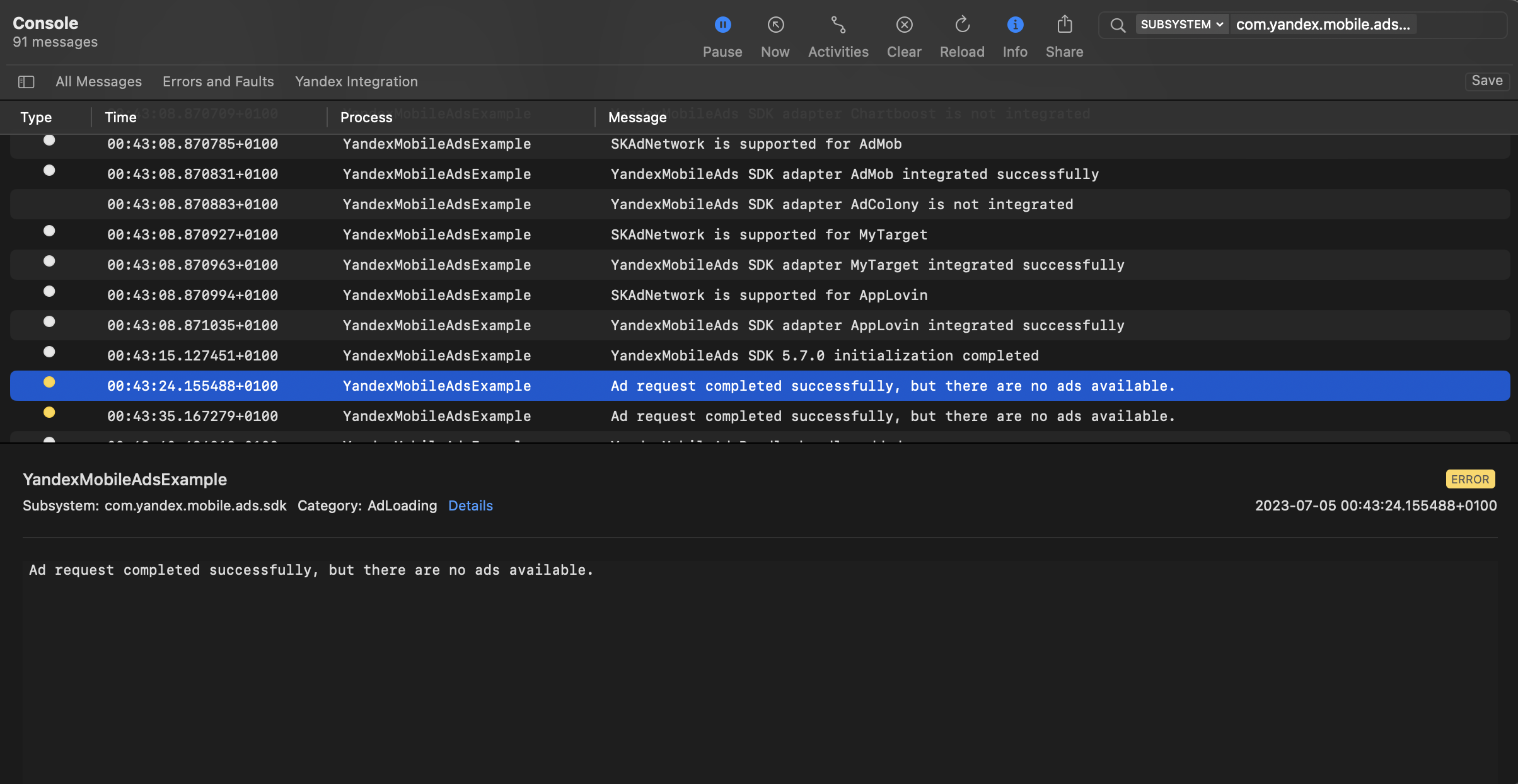
Recommendations
-
We don't recommend showing App Open Ads before the app reaches the splash screen.
Showing the splash screen enhances the user experience, making it more intuitive. This way, the user will know that they opened the right app and won't be surprised or confused by the ad. On this screen, you can also warn users about the upcoming ad. To do this, use a loading indicator or a simple text message informing the user that they can continue viewing the app content after the ad.
-
If there's a delay between the ad request and the impression, the user may briefly open your app and later see an unexpected ad that is unrelated to the app content. This can negatively impact the user experience, so it's best to avoid such situations. One solution is to show the splash screen before displaying the main app content and to begin ad impressions from that screen. We don't recommend displaying an ad if the app has already opened content after the splash screen.
-
Wait for new users to open the app and use it a few times before starting to serve App Open Ad impressions. Show the ad only to users who meet specific criteria (for example, if they completed a particular level, opened the app a certain number of times, or don't participate in reward offers). We don't recommend displaying an ad immediately after the app is installed.
-
Adjust the frequency of impressions based on user behavior. We don't recommend serving an ad at every cold or hot app start.
-
Display ads only if the app has been running in the background for a certain time (for example, 30 seconds, 2 minutes, or 15 minutes).
-
Be sure to conduct tests, because each app is unique and requires its own approach to maximize revenue without sacrificing user retention or time spent in the app. User behavior and engagement may change over time, so we recommend periodically testing different display strategies for App Open Ads within your app.
Additional resources
-
Link to GitHub.