Interstitial
Interstitial advertising is a full-screen ad format embedded within the app content during natural pauses, such as transitioning between game levels or completing a target action.
When an app displays an interstitial ad, the user can either click through to the advertiser's site or close the ad and return to the app.
During interstitial ad impressions, the user's attention is fully focused on the ad, which results in a higher cost for such impressions.
Appearance
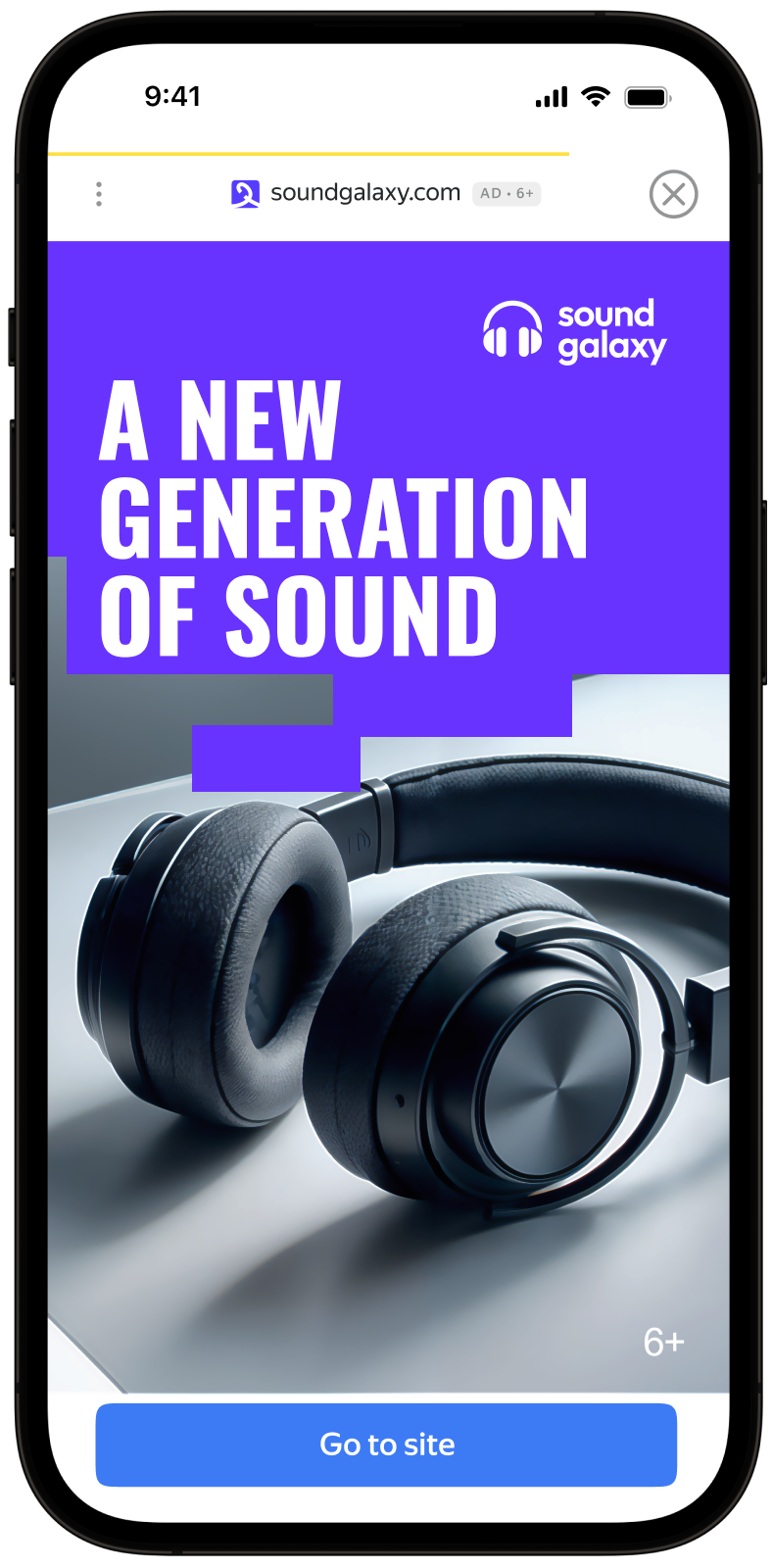
This guide covers the process of integrating interstitial ads into React Native apps. Besides code samples and instructions, it also contains format-specific recommendations and links to additional resources.
Prerequisite
- Follow the Yandex Mobile Ads React Native plugin integration steps described under Quick start.
- Make sure that you have the latest version of the Yandex Mobile Ads React Native plugin. If you're using mediation, update to the most recent single build version.
Implementation
Key steps for integrating interstitial ads:
- Create an
InterstitialAdLoader
. - Set up the parameters for loading ads using an
AdRequestConfiguration
object. - Load the
InterstitialAd
object. - Set the ad's callback functions.
- Display the
InterstitialAd
object.
Features of interstitial ad integration
- If the
loadAd
method returns an error, don't try to load a new ad again. If there's no other option, limit the number of ad load retries. This will help avoid constant unsuccessful requests and connection issues if there are limitations.
Loading ads
To load your interstitial ads, create an InterstitialAdLoader
object. To do this, call the InterstitialAdLoader.create()
method.
To create an AdRequestConfiguration
class instance, you'll need the placement ID from the Partner Interface (adUnitId).
You can also expand ad request parameters by providing information about the user's interests, page context, location, and other additional data when creating the AdRequestConfiguration
object. Extra context added to ad requests can greatly improve the ad quality. Read more in the Ad Targeting section.
To load your interstitial ad, call the loadAd
method of the InterstitialAdLoader
class object.
The example below shows how to load an interstitial ad:
let loader = await InterstitialAdLoader.create()
.catch((error) => {
// Handle error gracefully
return;
});
if (!loader) {
return;
}
let adRequestConfiguration = new AdRequestConfiguration(
'R-M-XXXXXX-Y', // for debug you can use 'demo-interstitial-yandex'
'20',
'context-query',
['context-tag'],
Gender.Female,
new Location(55.734202, 37.588063)
);
let ad = await loader.loadAd(adRequestConfiguration)
.then((ad) => {
// Ad loaded successfully
return ad;
})
.catch((error) => {
// Handle error gracefully
return;
});
Displaying ads
Interstitial ads should be displayed during natural pauses in the app's usage. A good example of this would be impressions between game levels or when a user completes an action, such as downloading a file.
To track an interstitial ad's lifecycle events, set callback functions for the InterstitialAd
class object.
To display an interstitial ad, use the show()
method:
ad.onAdShown = () => {
console.log('Did show');
};
ad.onAdFailedToShow = (error) => {
console.log(`Did fail to show with error: ${JSON.stringify(error)}`);
};
ad.onAdClicked = () => {
console.log('Did click');
};
ad.onAdDismissed = () => {
console.log('Did dismiss');
};
ad.onAdImpression = (impressionData) => {
console.log(`Did track impression: ${JSON.stringify(impressionData)}`);
};
ad.show();
Testing interstitial ad integration
Using demo ad units for ad testing
We recommend using test ads to test your interstitial ad integration and your app itself.
For guaranteed return of test ads for every ad request, we have created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-interstitial-yandex
.
Warning
Make sure that before placing the application in the store, you replaced the demo ad placement ID with a real one, obtained in Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can check your interstitial ad integration using the SDK's built-in analyzer.
The tool makes sure your interstitial ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool used for Android application debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you will see the following message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type interstitital was integrated successfully
If you're having problems integrating interstitial ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-interstitial-yandex
.
Warning
Make sure that before placing the application in the store, you replaced the demo ad placement ID with a real one, obtained in Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set sybsystem = com.yandex.mobile.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
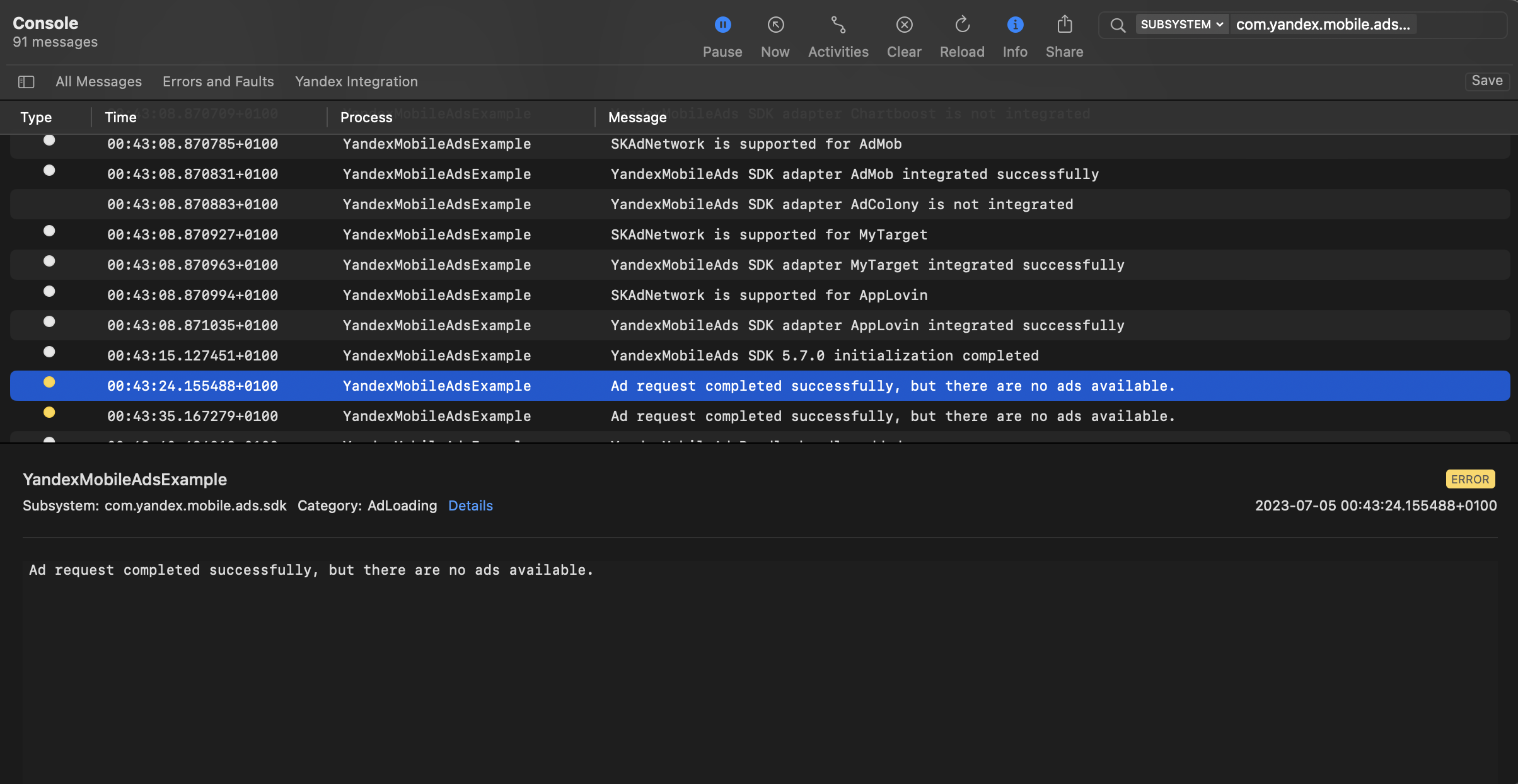
Tips
Ad preloading
Loading an ad may take several seconds, depending on the number of ad networks connected in mobile mediation and the user's internet speed. We recommend preloading ads before displaying them.
Call the loadAd
method in advance to instantly show the ad when it's needed.
To begin preloading the next ad immediately after serving the current one, you can link this process to the onAdDismissed
event.
If you cache ads on too many screens that are unlikely to be shown, your ad effectiveness could drop. For example, if users complete 2-3 game levels per session, you shouldn't cache ads for 6-7 screens. Your ad viewability could decrease otherwise, and the advertising system might deprioritize your app.
To make sure you're finding a good balance for your app, track the "Share of impressions" or "Share of visible impressions" metric in the partner interface. If it's under 20%, you should probably revise your caching algorithm. The higher the percentage of impressions, the better.
Additional resources
-
Link to GitHub.