Adaptive inline banner
Adaptive inline banners are a flexible format of banner advertising, providing maximum efficiency by optimizing the size of the ad on each device.
This ad type lets developers set a maximum allowable width and height for the ad, though the optimal ad size is still determined automatically. To select the best ad size, built-in adaptive banners use the maximum height rather than the fixed height. That provides room for improving performance.
Typically, that format is used in feed-based apps or contexts where it's okay to focus primarily on the ad.
This guide shows how to integrate adaptive inline banners into Unity apps. In addition to code examples and instructions, it has offers format-specific recommendations and links to additional resources.
Prerequisite
- Follow the process in Quick start for integrating the Yandex Mobile Ads Unity Plugin.
- Make sure you're running the latest Yandex Mobile Ads Unity Plugin version. If you're using mediation, make sure you're also running the latest version of the unified build.
Implementation
Key steps for integrating adaptive inline banners:
- Create and configure the Banner ad object.
- Register a callback method listener.
- Load the ad.
- Pass additional settings if you're using Adfox.
Features of adaptive inline banner integration
-
If you received an error in the
onAdFailedToLoad
event, don't try to load a new ad again. If there's no other option, limit the number of ad load retries. That will help avoid constant unsuccessful requests and connection issues when limitations arise. -
To ensure that adaptive inline banners function correctly, make your app layouts adaptive. Otherwise, your ads might render incorrectly.
-
Adaptive inline banners work best when using all the available width. In most cases, that's the full width of the device screen. Make sure to include all padding and safe display areas applicable to your app.
-
Adaptive inline banners are designed for placement in scrollable content. It can have the same height as the device screen or be limited to the maximum height depending on the API.
-
To get the size of the ad, use the
BannerAdSize.InlineSize(screenWidth, maxAdHeight)
method, which accepts the available width of the ad container and the maximum acceptable ad height as arguments. -
The
BannerAdSize
object, which is calculated using theBannerAdSize.InlineSize(screenWidth, maxAdHeight)
method, contains technical data for selecting the most effective ad sizes on the backend. The height of the ad may change every time it's loaded. The actual width and height of the ad are available after receiving a successful ad load message.
Adding Banner to the project
To display a banner in your app, create a Banner
object in the script (in C#) that is attached to the GameObject
.
using UnityEngine;
using YandexMobileAds;
using YandexMobileAds.Base;
public class YandexMobileAdsInlineBannerDemoScript : MonoBehaviour
{
private Banner banner;
private int GetScreenWidthDp()
{
int screenWidth = (int)Screen.safeArea.width;
return ScreenUtils.ConvertPixelsToDp(screenWidth);
}
private void RequestInlineBanner()
{
string adUnitId = "demo-banner-yandex"; // замените на "R-M-XXXXXX-Y"
BannerAdSize bannerMaxSize = BannerAdSize.InlineSize(GetScreenWidthDp(), 100);
banner = new Banner(adUnitId, bannerMaxSize, AdPosition.BottomCenter);
}
}
The Banner
constructor contains the following parameters:
-
AdUnitId
: A unique identifier that is issued in the Partner interface and looks like this: R-M-XXXXXX-Y.Tip
For testing purposes, you can use the demo unit ID: "demo-banner-yandex". Before publishing your ad, make sure you replace the demo unit ID with a real ad unit ID.
-
BannerAdSize
: Size of the banner you want to display. -
AdPosition
: The position on the screen. -
InlineSize(int width, int maxHeight)
: Banner dimensions.
Set the width and maximum allowable height. The ad will fit into these dimensions. The height of the selected ad won't exceed the height of the device screen.
Loading and rendering ads
After creating and configuring an object of the Banner
class, you need to load the ad. To load an ad, use the LoadAd
method, which takes the AdRequest
object as an argument.
The ad size must also be calculated for each device before loading adaptive inline banners.
The operation is performed automatically via the SDK API: BannerAdSize.InlineSize(screenWidth, maxAdHeight)
.
You can expand the ad request parameters through AdRequest.Builder()
by passing user interests, contextual app data, location details, or other data in the request. Delivering additional contextual data in the request can significantly improve your ad quality.
To notify when ads load or fail to load and to track the life cycle of adaptive inline banners, set callback functions for the BannerAd
class object.
private void RequestInlineBanner()
{
// ...
AdRequest request = new AdRequest.Builder().Build();
banner.LoadAd(request);
}
Banner ad events
To track events that occur in banner ads, register a delegate for the appropriate EventHandler
, as shown below:
using System;
// ...
private void RequestInlineBanner()
{
// ...
// Called when a rewarded ad is loaded
banner.OnAdLoaded += HandleAdLoaded;
// Called when there was an error loading the ad
banner.OnAdFailedToLoad += HandleAdFailedToLoad;
// Called when the app goes inactive because the user tapped an ad and is about to switch to a different app (for example, a browser).
banner.OnLeftApplication += HandleLeftApplication;
// Called when the user returns to the app after a tap
banner.OnReturnedToApplication += HandleReturnedToApplication;
// Called when the user taps the ad
banner.OnAdClicked += HandleAdClicked;
// Called when an impression is registered
banner.OnImpression += HandleImpression;
// ...
}
private void HandleAdLoaded(object sender, EventArgs args)
{
Debug.Log("AdLoaded event received");
banner.Show();
}
private void HandleAdFailedToLoad(object sender, AdFailureEventArgs args)
{
Debug.Log($"AdFailedToLoad event received with message: {args.Message}");
// We strongly advise against loading a new ad using this method
}
private void HandleLeftApplication(object sender, EventArgs args)
{
Debug.Log("LeftApplication event received");
}
private void HandleReturnedToApplication(object sender, EventArgs args)
{
Debug.Log("ReturnedToApplication event received");
}
private void HandleAdClicked(object sender, EventArgs args)
{
Debug.Log("AdClicked event received");
}
private void HandleImpression(object sender, ImpressionData impressionData)
{
var data = impressionData == null ? "null" : impressionData.rawData;
Debug.Log($"HandleImpression event received with data: {data}");
}
Testing adaptive inline banner integration
Using demo ad units for ad testing
We recommend using test ads to test your adaptive inline banner integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-banner-yandex
.
Warning
Before publishing your app in the store, make sure to replace the demo ad placement ID with a real one obtained from the Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your adaptive inline banner integration using the SDK's built-in analyzer.
The tool makes sure your ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool for Android app debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you'll see this message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type banner was integrated successfully
If you're having problems integrating banner ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-banner-yandex
.
Warning
Before publishing your app to the store, make sure you replace the demo ad unit ID with a real one obtained from the Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set sybsystem = com.yandex.mobile.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
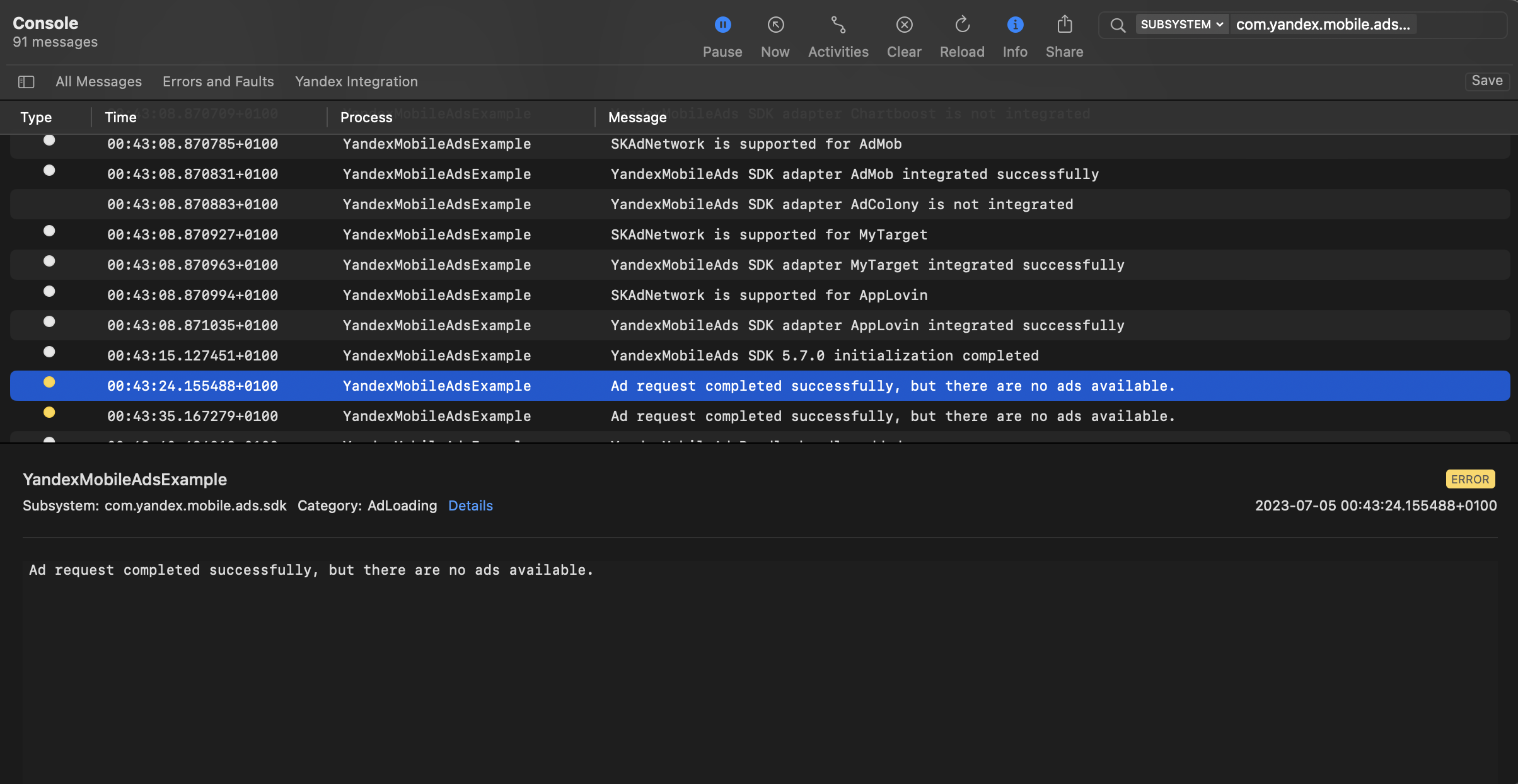
Tips
Ad preloading
Loading an ad may take several seconds, depending on the number of ad networks connected in mobile mediation and the user's internet speed. We recommend preloading ads before displaying them.
You can load an ad at app startup or just before it's shown. Make sure to preserve the object between scenes using the DontDestroyOnLoad(gameObject)
call. That ensures the ad that's loaded is not deleted with the gameObject
on scene change.
When you then need to render the banner, call banner.Show()
.
If you cache ads on too many screens that are unlikely to be shown, your ad effectiveness could drop. For example, if users complete 2-3 game levels per session, you shouldn't cache ads for 6-7 screens. Your ad viewability could decrease otherwise, and the advertising system might deprioritize your app.
To make sure you're finding a good balance for your app, track the "Share of impressions" or "Share of visible impressions" metric in the partner interface. If it's under 20%, you should probably revise your caching algorithm. The higher the percentage of impressions, the better.
Clearing unused memory
We recommend calling banner?.Destroy()
and re-creating the Banner
object before each ad request. That ensures that all memory that was utilized is cleared, and the app can request and show ads many times without overwhelming the device. That can also prevent code errors related to repeated ad loading and lifecycle disruptions.
Additional resources
-
Link to GitHub.