Rewarded ads
Rewarded ads are a popular full-screen ad format, where the user receives a reward for viewing the ad.
The display of this ad type is activated by the user, for instance, to receive a bonus or additional life in a game.
High user motivation makes this ad format the most popular and profitable in free apps.
Appearance
This guide will show how to integrate rewarded ads into Unity apps. In addition to code examples and instructions, it contains format-specific recommendations and links to additional resources.
Prerequisite
- Follow the process in Quick start for integrating the Yandex Mobile Ads Unity Plugin.
- Make sure you're running the latest Yandex Mobile Ads Unity Plugin version. If you're using mediation, make sure you're also running the latest version of the unified build.
Implementation
Key steps for integrating rewarded ads:
- Create a
RewardedAdLoader
ad loader and register the listeners for ad loading events. - Set the ad loading parameters in
AdRequestConfiguration
. - Load the ad using the
RewardedAdLoader.LoadAd(AdRequestConfiguration)
method. - Register listeners for events where users interact with your ad.
- Show the ad by calling the
RewardedAd.Show()
method. - Release the resources
Features of rewarded ad integration
-
If you received an error in the
onAdFailedToLoad
event, don't try to load a new ad again. If there's no other option, limit the number of ad load retries. That will help avoid constant unsuccessful requests and connection issues when limitations arise. -
We recommend using a single instance of
RewardedAdLoader
for all ad loads to improve performance.
Step-by-step guide
-
Create a
RewardedAdLoader
object in the C# script attached toGameObject
, and then register the ad load event listeners.using UnityEngine; using YandexMobileAds; using YandexMobileAds.Base; public class YandexMobileAdsRewardedAdDemoScript : MonoBehaviour { private RewardedAdLoader rewardedAdLoader; private RewardedAd rewardedAd; private void SetupLoader() { rewardedAdLoader = new RewardedAdLoader(); rewardedAdLoader.OnAdLoaded += HandleAdLoaded; rewardedAdLoader.OnAdFailedToLoad += HandleAdFailedToLoad; // ... } public void HandleAdLoaded(object sender, RewardedAdLoadedEventArgs args) { // Rewarded ad was loaded successfully. Now you can handle it. rewardedAd = args.RewardedAd; } public void HandleAdFailedToLoad(object sender, AdFailedToLoadEventArgs args) { // Ad {args.AdUnitId} failed for to load with {args.Message} // Attempting to load new ad from the OnAdFailedToLoad event is strongly discouraged. } }
-
Set up the ad loading parameters in
AdRequestConfiguration
.string adUnitId = "demo-rewarded-yandex"; // replace with "R-M-XXXXXX-Y" AdRequestConfiguration adRequestConfiguration = new AdRequestConfiguration.Builder(adUnitId).Build();
AdUnitId
: A unique identifier that is issued in the Partner interface and looks like this: R-M-XXXXXX-Y.Tip
For testing purposes, you can use the demo unit ID: "demo-rewarded-yandex". Before publishing your ad, make sure to replace the demo unit ID with a real ad unit ID.
You can expand the ad request parameters through
AdRequestConfiguration.Builder
by passing user interests, contextual app data, location details, or other data. Delivering additional contextual data in the request can significantly improve your ad quality. -
Load the ad with the
LoadAd
method, passingAdRequestConfiguration
as an argument.rewardedAdLoader.LoadAd(adRequestConfiguration);
-
Register listeners for events where users interact with your ad.
using System; // ... rewardedAd.OnAdClicked += HandleAdClicked; rewardedAd.OnAdShown += HandleAdShown; rewardedAd.OnAdFailedToShow += HandleAdFailedToShow; rewardedAd.OnAdImpression += HandleImpression; rewardedAd.OnAdDismissed += HandleAdDismissed; rewardedAd.OnRewarded += HandleRewarded; // ... public void HandleAdClicked(object sender, EventArgs args) { // Called when a click is recorded for rewarded ad. } public void HandleAdShown(object sender, EventArgs args) { // Called when an ad is shown. } public void HandleAdFailedToShow(object sender, AdFailureEventArgs args) { // Called when an ad failed to show. } public void HandleAdDismissed(object sender, EventArgs args) { // Called when an ad is dismissed. } public void HandleImpression(object sender, ImpressionData impressionData) { // Called when an impression is recorded for an ad. } public void HandleRewarded(object sender, Reward args) { // Called when the user can be rewarded with {args.type} and {args.amount}. }
-
Show the ad by calling
Show()
on theRewardedAd
object.private void ShowRewardedAd() { if (rewardedAd != null) { rewardedAd.Show(); } }
-
Call
Destroy()
on ads that are shown and clear links that are no longer used on the current screen.That releases the resources and prevents memory leaks.
public void DestroyRewardedAd() { if (rewardedAd != null) { rewardedAd.Destroy(); rewardedAd = null; } }
Testing the rewarded ad integration
Using demo ad units for ad testing
We recommend using test ads to test your rewarded ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-rewarded-yandex
.
Warning
Before publishing your app in the store, make sure to replace the demo ad placement ID with a real one obtained from the Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your rewarded ad integration using the SDK's built-in analyzer.
The tool checks makes sure your rewarded ads are integrated properly and outputs a detailed report to the log. To view the report, search for the "YandexAds" keyword in the Logcat tool for Android app debugging.
adb logcat -v brief '*:S YandexAds'
If the integration is successful, you'll see this message:
adb logcat -v brief '*:S YandexAds'
mobileads$ adb logcat -v brief '*:S YandexAds'
I/YandexAds(13719): [Integration] Ad type rewarded was integrated successfully
If you're having problems integrating rewarded ads, you'll get a detailed report on the issues and recommendations for how to fix them.
Using demo ad units for ad testing
We recommend using test ads to test your ad integration and your app itself.
To guarantee that test ads are returned for every ad request, we created a special demo ad placement ID. Use it to check your ad integration.
Demo adUnitId: demo-rewarded-yandex
.
Warning
Before publishing your app to the store, make sure you replace the demo ad unit ID with a real one obtained from the Partner Interface.
You can find the list of available demo ad placement IDs in the Demo ad units for testing section.
Testing ad integration
You can test your ad integration using the native Console tool.
To view detailed logs, call the YMAMobileAds
class's enableLogging
method.
YMAMobileAds.enableLogging()
To view SDK logs, go to the Console tool and set Subsystem = com.mobile.ads.ads.sdk
. You can also filter logs by category and error level.
If you're having problems integrating ads, you'll get a detailed report on the issues and recommendations for how to fix them.
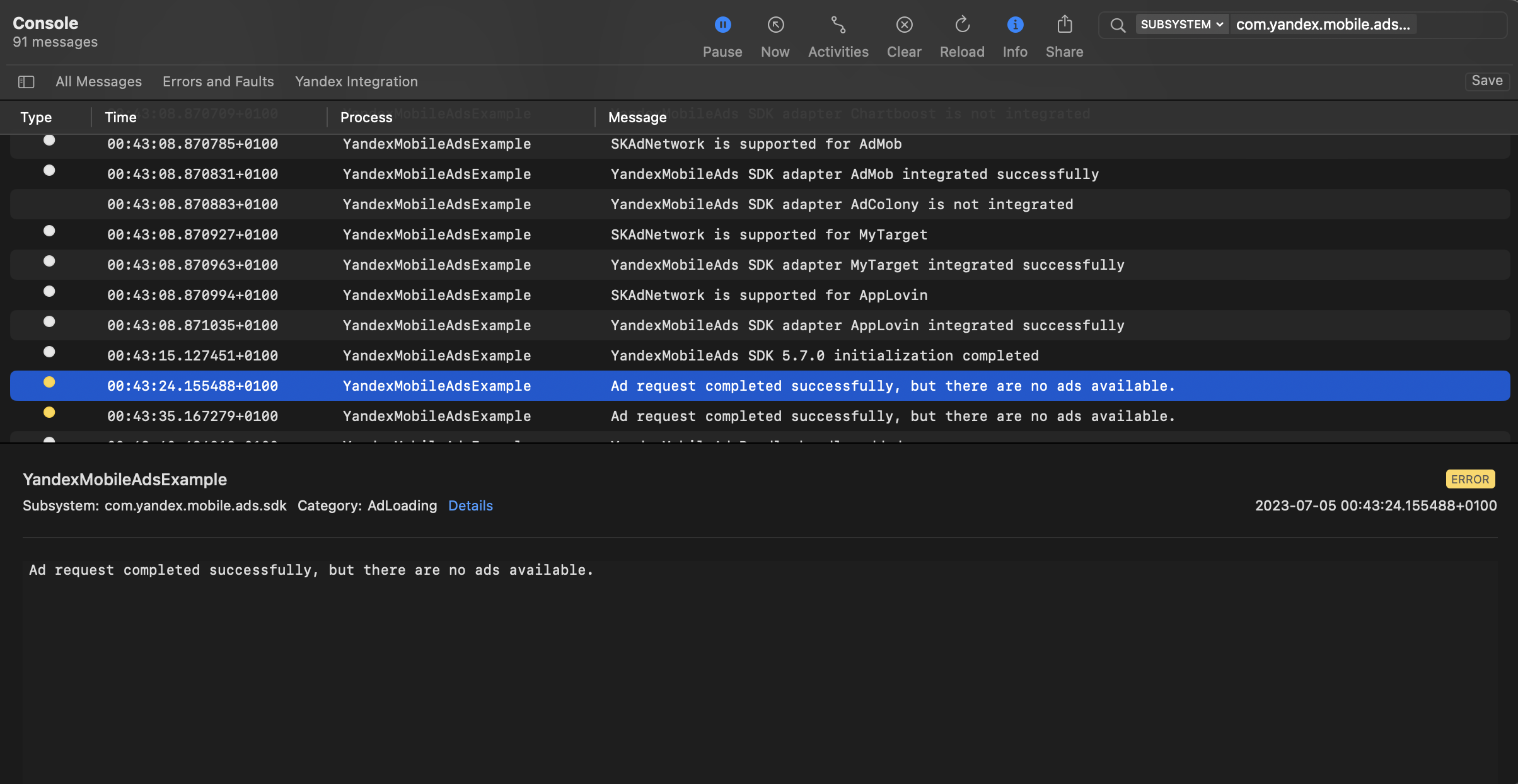
Tips
Ad preloading
Loading an ad may take several seconds, depending on the number of ad networks connected in mobile mediation and the user's internet speed. We recommend preloading ads before displaying them.
You can call ad loading in the Awake
method for gameObject
. Make sure to preserve the object between scenes using the DontDestroyOnLoad()
call. That ensures the ad that's loaded is not deleted with gameObject
on scene change.
private void Awake()
{
SetupLoader();
RequestRewardedAd();
DontDestroyOnLoad(gameObject);
}
Along with that, you can bind loading of the next ad to the callback functions run when ad show completes or fails. For example, like this:
public void HandleAdFailedToShow(object sender, EventArgs args)
{
// Called when an ad failed to show.
// Clear resources after an ad dismissed.
DestroyRewardedAd();
// Now you can preload the next rewarded ad.
RequestRewardedAd();
}
public void HandleAdDismissed(object sender, EventArgs args)
{
// Called when an ad is dismissed.
// Clear resources after an ad dismissed.
DestroyRewardedAd();
// Now you can preload the next rewarded ad.
RequestRewardedAd();
}
If you cache ads on too many screens that are unlikely to be shown, your ad effectiveness could drop. For example, if users complete 2-3 game levels per session, you shouldn't cache ads for 6-7 screens. Your ad viewability could decrease otherwise, and the advertising system might deprioritize your app.
To make sure you're finding a good balance for your app, track the "Share of impressions" or "Share of visible impressions" metric in the partner interface. If it's under 20%, you should probably revise your caching algorithm. The higher the percentage of impressions, the better.
Full code example
using System;
using UnityEngine;
using UnityEngine.UI;
using YandexMobileAds;
using YandexMobileAds.Base;
public class YandexMobileAdsRewardedAdDemoScript : MonoBehaviour
{
private RewardedAdLoader rewardedAdLoader;
private RewardedAd rewardedAd;
[SerializeField] private Button button;
private void Awake()
{
SetupLoader();
RequestRewardedAd();
DontDestroyOnLoad(gameObject);
button = this.GetComponent<Button>();
button.onClick.AddListener(ShowRewardedAd);
}
private void SetupLoader()
{
rewardedAdLoader = new RewardedAdLoader();
rewardedAdLoader.OnAdLoaded += HandleAdLoaded;
rewardedAdLoader.OnAdFailedToLoad += HandleAdFailedToLoad;
}
private void RequestRewardedAd()
{
string adUnitId = "demo-rewarded-yandex"; // replace with "R-M-XXXXXX-Y"
AdRequestConfiguration adRequestConfiguration = new AdRequestConfiguration.Builder(adUnitId).Build();
rewardedAdLoader.LoadAd(adRequestConfiguration);
}
private void ShowRewardedAd()
{
if (rewardedAd != null)
{
rewardedAd.Show();
}
}
public void HandleAdLoaded(object sender, RewardedAdLoadedEventArgs args)
{
// The ad was loaded successfully. Now you can handle it.
rewardedAd = args.RewardedAd;
// Add events handlers for ad actions
rewardedAd.OnAdClicked += HandleAdClicked;
rewardedAd.OnAdShown += HandleAdShown;
rewardedAd.OnAdFailedToShow += HandleAdFailedToShow;
rewardedAd.OnAdImpression += HandleImpression;
rewardedAd.OnAdDismissed += HandleAdDismissed;
rewardedAd.OnRewarded += HandleRewarded;
}
public void HandleAdFailedToLoad(object sender, AdFailedToLoadEventArgs args)
{
// Ad {args.AdUnitId} failed for to load with {args.Message}
// Attempting to load a new ad from the OnAdFailedToLoad event is strongly discouraged.
}
public void HandleAdDismissed(object sender, EventArgs args)
{
// Called when an ad is dismissed.
// Clear resources after an ad dismissed.
DestroyRewardedAd();
// Now you can preload the next rewarded ad.
RequestRewardedAd();
}
public void HandleAdFailedToShow(object sender, AdFailureEventArgs args)
{
// Called when rewarded ad failed to show.
// Clear resources after an ad dismissed.
DestroyRewardedAd();
// Now you can preload the next rewarded ad.
RequestRewardedAd();
}
public void HandleAdClicked(object sender, EventArgs args)
{
// Called when a click is recorded for an ad.
}
public void HandleAdShown(object sender, EventArgs args)
{
// Called when an ad is shown.
}
public void HandleImpression(object sender, ImpressionData impressionData)
{
// Called when an impression is recorded for an ad.
}
public void HandleRewarded(object sender, Reward args)
{
// Called when the user can be rewarded with {args.type} and {args.amount}.
}
public void DestroyRewardedAd()
{
if (rewardedAd != null)
{
rewardedAd.Destroy();
rewardedAd = null;
}
}
}
Additional resources
-
Link to GitHub.